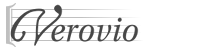 |
Verovio
Source code documentation
|
8 #ifndef __VRV_ADJUSTFLOATINGPOSITIONERFUNCTOR_H__
9 #define __VRV_ADJUSTFLOATINGPOSITIONERFUNCTOR_H__
41 FunctorCode VisitStaffAlignment(
StaffAlignment *staffAlignment)
override;
42 FunctorCode VisitSystem(
System *system)
override;
84 void SetClassIDs(
const std::vector<ClassId> &classIds) { m_classIds = classIds; }
85 void SetPlace(data_STAFFREL place) { m_place = place; }
92 FunctorCode VisitStaffAlignment(StaffAlignment *staffAlignment)
override;
100 void AdjustGroupsMonotone(
const StaffAlignment *staffAlignment,
const ArrayOfFloatingPositioners &positioners,
101 ArrayOfIntPairs &grpIdYRel)
const;
107 std::vector<ClassId> m_classIds;
109 data_STAFFREL m_place;
138 FunctorCode VisitStaffAlignment(
StaffAlignment *staffAlignment)
override;
139 FunctorCode VisitSystem(
System *system)
override;
155 #endif // __VRV_ADJUSTFLOATINGPOSITIONERFUNCTOR_H__
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class adjusts the position of all floating positioners, staff by staff.
Definition: adjustfloatingpositionerfunctor.h:22
This class adjusts the position of all floating positioners that are grouped, staff by staff.
Definition: adjustfloatingpositionerfunctor.h:65
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: adjustfloatingpositionerfunctor.h:35
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: adjustfloatingpositionerfunctor.h:132
This class represents a system in a laid-out score (Doc).
Definition: system.h:36
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: adjustfloatingpositionerfunctor.h:78
This class stores an alignement position staves will point to.
Definition: verticalaligner.h:172
This abstract class is the base class for all mutable functors that need access to the document.
Definition: functor.h:151
This class adjusts the position of floating positioners placed between staves.
Definition: adjustfloatingpositionerfunctor.h:119