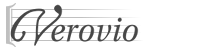 |
Verovio
Source code documentation
|
8 #ifndef __VRV_ADJUSTTUPLETSYFUNCTOR_H__
9 #define __VRV_ADJUSTTUPLETSYFUNCTOR_H__
41 FunctorCode VisitTuplet(
Tuplet *tuplet)
override;
50 void AdjustTupletBracketY(
Tuplet *tuplet,
const Staff *staff)
const;
52 void AdjustTupletNumY(
Tuplet *tuplet,
const Staff *staff)
const;
61 int CalcBracketShift(
Point referencePos,
double slope,
int sign,
const std::list<Point> &obstacles)
const;
83 const TupletNum *tupletNum,
const Staff *staff, data_STAFFREL_basic drawingNumPos,
int drawingY);
96 void SetHorizontalMargin(
int margin) { m_horizontalMargin = margin; }
97 void SetVerticalMargin(
int margin) { m_verticalMargin = margin; }
103 int GetDrawingY()
const {
return m_drawingY; }
109 FunctorCode VisitLayerElement(
const LayerElement *layerElement)
override;
120 const TupletNum *m_tupletNum;
122 data_STAFFREL_basic m_drawingNumPos;
124 int m_horizontalMargin;
125 int m_verticalMargin;
127 const Staff *m_staff;
158 FunctorCode VisitTuplet(
Tuplet *tuplet)
override;
173 #endif // __VRV_ADJUSTTUPLETSYFUNCTOR_H__
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class models a bracket as a layer element part and has no direct MEI equivalent.
Definition: elementpart.h:150
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: adjusttupletsyfunctor.h:152
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: adjusttupletsyfunctor.h:35
This class models a tuplet num as a layer element part and has no direct MEI equivalent.
Definition: elementpart.h:249
This abstract class is the base class for all const functors.
Definition: functor.h:126
This class adjusts the Y position of tuplets with inner slurs.
Definition: adjusttupletsyfunctor.h:139
This class calculates the Y position of tuplet brackets and num.
Definition: adjusttupletsyfunctor.h:22
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: adjusttupletsyfunctor.h:90
Simple class for representing points.
Definition: devicecontextbase.h:203
This abstract class is the base class for all mutable functors that need access to the document.
Definition: functor.h:151
This class calculates the Y relative position of tupletNum based on overlaps with other elements.
Definition: adjusttupletsyfunctor.h:76