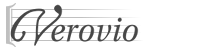 |
Verovio
Source code documentation
|
8 #ifndef __VRV_ALIGNFUNCTOR_H__
9 #define __VRV_ALIGNFUNCTOR_H__
23 const Mensur *mensur = NULL;
26 data_DURATION equivalence = DURATION_brevis;
58 FunctorCode VisitLayer(
Layer *layer)
override;
59 FunctorCode VisitLayerEnd(
Layer *layer)
override;
60 FunctorCode VisitLayerElement(
LayerElement *layerElement)
override;
61 FunctorCode VisitMeasure(
Measure *measure)
override;
62 FunctorCode VisitMeasureEnd(
Measure *measure)
override;
63 FunctorCode VisitMeterSigGrp(
MeterSigGrp *meterSigGrp)
override;
64 FunctorCode VisitStaff(
Staff *staff)
override;
65 FunctorCode VisitSystem(
System *system)
override;
82 data_NOTATIONTYPE m_notationType;
84 ElementScoreDefRole m_scoreDefRole;
86 bool m_isFirstMeasure;
88 bool m_hasMultipleLayer;
117 void StoreCastOffSystemWidths(
bool storeWidths) { m_storeCastOffSystemWidths = storeWidths; }
123 FunctorCode VisitDiv(Div *div)
override;
124 FunctorCode VisitMeasure(Measure *measure)
override;
125 FunctorCode VisitScoreDef(ScoreDef *scoreDef)
override;
126 FunctorCode VisitSection(Section *section)
override;
127 FunctorCode VisitSystem(System *system)
override;
128 FunctorCode VisitSystemEnd(System *system)
override;
141 int m_justifiableWidth;
143 bool m_applySectionRestartShift;
145 bool m_storeCastOffSystemWidths;
175 FunctorCode VisitDiv(
Div *div)
override;
176 FunctorCode VisitFig(
Fig *fig)
override;
177 FunctorCode VisitMeasure(
Measure *measure)
override;
178 FunctorCode VisitPageEnd(
Page *page)
override;
179 FunctorCode VisitRend(
Rend *rend)
override;
180 FunctorCode VisitRunningElement(
RunningElement *runningElement)
override;
181 FunctorCode VisitStaff(
Staff *staff)
override;
182 FunctorCode VisitStaffAlignmentEnd(
StaffAlignment *staffAlignment)
override;
183 FunctorCode VisitSyllable(
Syllable *syllable)
override;
184 FunctorCode VisitSystem(
System *system)
override;
185 FunctorCode VisitSystemEnd(
System *system)
override;
186 FunctorCode VisitVerse(
Verse *verse)
override;
203 int m_cumulatedShift;
205 int m_justificationSum;
236 void SetShift(
int shift) { m_shift = shift; }
237 void SetSystemSpacing(
int spacing) { m_systemSpacing = spacing; }
244 FunctorCode VisitPage(Page *page)
override;
245 FunctorCode VisitPageEnd(Page *page)
override;
246 FunctorCode VisitSystem(System *system)
override;
261 int m_prevBottomOverflow;
262 int m_prevBottomClefOverflow;
264 double m_justificationSum;
269 #endif // __VRV_ALIGNFUNCTOR_H__
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class represents a measure in a page-based score (Doc).
Definition: measure.h:37
This class models the MEI <mensur> element.
Definition: syllable.h:25
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class models the MEI <meterSig> element.
Definition: metersig.h:27
Regroup pointers to meterSig, mensur and proport objects.
Definition: alignfunctor.h:21
This class represents an MEI Div.
Definition: div.h:24
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: alignfunctor.h:52
This class represents a MEI meterSigGrp.
Definition: metersiggrp.h:28
Definition: fraction.h:19
This class models the MEI <mensur> element.
Definition: mensur.h:27
This class aligns the measures by adjusting the m_drawingXRel position looking at the MeasureAligner.
Definition: alignfunctor.h:99
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: alignfunctor.h:112
This class represents running elements (headers and footers).
Definition: runningelement.h:28
This class models the MEI <fig> element.
Definition: fig.h:23
This class aligns horizontally the content of a page.
Definition: alignfunctor.h:39
This class models the MEI <proport> element.
Definition: proport.h:23
This class represents a page in a laid-out score (Doc).
Definition: page.h:31
This class aligns the content of a measure It contains a vector of Alignment.
Definition: horizontalaligner.h:425
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: alignfunctor.h:169
This class represents a system in a laid-out score (Doc).
Definition: system.h:36
This class aligns the system by adjusting the m_drawingYRel position looking at the SystemAligner.
Definition: alignfunctor.h:217
This class stores an alignement position staves will point to.
Definition: verticalaligner.h:172
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
This class represents a layer in a laid-out score (Doc).
Definition: layer.h:33
This class models the MEI <rend> element.
Definition: rend.h:25
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: alignfunctor.h:230
This class aligns the content of a system It contains a vector of StaffAlignment.
Definition: verticalaligner.h:31
This abstract class is the base class for all mutable functors that need access to the document.
Definition: functor.h:151
This class vertically aligns the content of a page.
Definition: alignfunctor.h:156