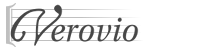 |
Verovio
Source code documentation
|
8 #ifndef __VRV_BOUNDING_BOX_H__
9 #define __VRV_BOUNDING_BOX_H__
17 #define BEZIER_APPROXIMATION 50.0
19 class BeamDrawingInterface;
21 class FloatingCurvePositioner;
41 virtual ClassId GetClassId()
const = 0;
42 bool Is(ClassId classId)
const {
return (this->GetClassId() == classId); }
43 bool Is(
const std::vector<ClassId> &classIds)
const;
49 virtual void UpdateContentBBoxX(
int x1,
int x2);
51 virtual void UpdateContentBBoxY(
int y1,
int y2);
52 virtual void UpdateSelfBBoxX(
int x1,
int x2);
53 virtual void UpdateSelfBBoxY(
int y1,
int y2);
56 bool HasSelfBB()
const;
57 bool HasSelfHorizontalBB()
const;
58 bool HasSelfVerticalBB()
const;
59 bool HasContentBB()
const;
60 bool HasContentHorizontalBB()
const;
61 bool HasContentVerticalBB()
const;
62 bool HasEmptyBB()
const;
70 char32_t GetBoundingBoxGlyph()
const {
return m_smuflGlyph; }
71 int GetBoundingBoxGlyphFontSize()
const {
return m_smuflGlyphFontSize; }
83 virtual int GetDrawingX()
const = 0;
85 virtual int GetDrawingY()
const = 0;
92 virtual void ResetCachedDrawingX()
const = 0;
94 virtual void ResetCachedDrawingY()
const = 0;
100 int GetSelfBottom()
const {
return (this->GetDrawingY() + m_selfBB_y1); }
102 int GetSelfTop()
const {
return (this->GetDrawingY() + m_selfBB_y2); }
103 int GetSelfLeft()
const {
return (this->GetDrawingX() + m_selfBB_x1); }
104 int GetSelfRight()
const {
return (this->GetDrawingX() + m_selfBB_x2); }
105 int GetContentBottom()
const {
return (this->GetDrawingY() + m_contentBB_y1); }
106 int GetContentTop()
const {
return (this->GetDrawingY() + m_contentBB_y2); }
107 int GetContentLeft()
const {
return (this->GetDrawingX() + m_contentBB_x1); }
108 int GetContentRight()
const {
return (this->GetDrawingX() + m_contentBB_x2); }
110 int GetSelfX1()
const {
return m_selfBB_x1; }
111 int GetSelfX2()
const {
return m_selfBB_x2; }
112 int GetSelfY1()
const {
return m_selfBB_y1; }
113 int GetSelfY2()
const {
return m_selfBB_y2; }
114 int GetContentX1()
const {
return m_contentBB_x1; }
115 int GetContentX2()
const {
return m_contentBB_x2; }
116 int GetContentY1()
const {
return m_contentBB_y1; }
117 int GetContentY2()
const {
return m_contentBB_y2; }
123 int GetBottomBy(Accessor type)
const {
return ((type == SELF) ? GetSelfBottom() : GetContentBottom()); }
125 int GetTopBy(Accessor type)
const {
return ((type == SELF) ? GetSelfTop() : GetContentTop()); }
126 int GetLeftBy(Accessor type)
const {
return ((type == SELF) ? GetSelfLeft() : GetContentLeft()); }
127 int GetRightBy(Accessor type)
const {
return ((type == SELF) ? GetSelfRight() : GetContentRight()); }
128 int GetX1By(Accessor type)
const {
return ((type == SELF) ? GetSelfX1() : GetContentX1()); }
129 int GetX2By(Accessor type)
const {
return ((type == SELF) ? GetSelfX2() : GetContentX2()); }
130 int GetY1By(Accessor type)
const {
return ((type == SELF) ? GetSelfY1() : GetContentY1()); }
131 int GetY2By(Accessor type)
const {
return ((type == SELF) ? GetSelfY2() : GetContentY2()); }
138 bool HorizontalContentOverlap(
const BoundingBox *other,
int margin = 0)
const;
140 bool VerticalContentOverlap(
const BoundingBox *other,
int margin = 0)
const;
141 bool HorizontalSelfOverlap(
const BoundingBox *other,
int margin = 0)
const;
142 bool VerticalSelfOverlap(
const BoundingBox *other,
int margin = 0)
const;
148 int HorizontalLeftOverlap(
const BoundingBox *other,
const Doc *doc,
int margin = 0,
int vMargin = 0)
const;
150 int HorizontalRightOverlap(
const BoundingBox *other,
const Doc *doc,
int margin = 0,
int vMargin = 0)
const;
151 int VerticalTopOverlap(
const BoundingBox *other,
const Doc *doc,
int margin = 0,
int hMargin = 0)
const;
152 int VerticalBottomOverlap(
const BoundingBox *other,
const Doc *doc,
int margin = 0,
int hMargin = 0)
const;
159 int GetCutOutTop(
const Resources &resources)
const;
161 int GetCutOutBottom(
const Resources &resources)
const;
162 int GetCutOutLeft(
const Resources &resources)
const;
163 int GetCutOutRight(
const Resources &resources)
const;
165 int GetCutOutLeft(
const Resources &resources,
bool fromTop)
const;
166 int GetCutOutRight(
const Resources &resources,
bool fromTop)
const;
186 bool fromBeamContentSide =
false)
const;
192 static std::pair<double, int> ApproximateBezierExtrema(
193 const Point bezier[4],
bool isMaxExtrema,
int approximationSteps = BEZIER_APPROXIMATION);
254 const Point bezier[4],
Point &pos,
int &width,
int &height,
int &minYPos,
int &maxYPos);
261 static int RectRightOverlap(
const Point rect1[2],
const Point rect2[2],
int margin,
int vMargin);
262 static int RectTopOverlap(
const Point rect1[2],
const Point rect2[2],
int margin,
int hMargin);
263 static int RectBottomOverlap(
const Point rect1[2],
const Point rect2[2],
int margin,
int hMargin);
279 int GetRectangles(
const SMuFLGlyphAnchor &anchor,
Point rect[2][2],
const Resources &resources)
const;
281 int GetRectangles(
const SMuFLGlyphAnchor &anchor1,
const SMuFLGlyphAnchor &anchor2,
Point rect[3][2],
289 bool GetGlyph2PointRectangles(
290 const SMuFLGlyphAnchor &anchor1,
const SMuFLGlyphAnchor &anchor2,
const Glyph *glyph1,
Point rect[3][2])
const;
296 bool GetGlyph1PointRectangles(
const SMuFLGlyphAnchor &anchor,
const Glyph *glyph,
Point rect[2][2])
const;
308 mutable int m_cachedDrawingY;
314 int m_contentBB_x1, m_contentBB_y1, m_contentBB_x2, m_contentBB_y2;
316 int m_selfBB_x1, m_selfBB_y1, m_selfBB_x2, m_selfBB_y2;
323 char32_t m_smuflGlyph;
328 int m_smuflGlyphFontSize;
351 bool IsEmpty()
const {
return (m_segments.empty()); }
371 void AddGap(
int start,
int end);
386 ArrayOfIntPairs m_segments;
static Point CalcPointAtBezier(const Point bezier[4], double t)
Calculate point (X,Y) coordinaties on the bezier curve.
static void CalcLinearInterpolation(Point &dest, const Point &a, const Point &b, double t)
Calculate linear interpolation between two points at time t.
static std::set< double > SolveCubicPolynomial(double a, double b, double c, double d)
Solve the cubic equation ax^3 + bx^2 + cx + d = 0 Returns up to three real roots.
static double CalcBezierParamAtPosition(const Point bezier[4], int x)
Calculate the t parameter of a bezier at position x.
bool IsUnsegmented() const
Check if the line is one single segment.
Definition: boundingbox.h:356
static double CalcSlope(const Point &p1, const Point &p2)
Calculate the slope represented by two points.
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
bool IsEmpty() const
Check if the segmented line is empty.
Definition: boundingbox.h:351
static double CalcDistance(const Point &p1, const Point &p2)
Calculate the euclidean distance between two points.
bool Encloses(const Point point) const
Return true if the bounding box encloses the point.
static int CalcBezierAtPosition(const Point bezier[4], int x)
Calculate the y position of a bezier at position x.
virtual void ResetBoundingBox()
Reset the bounding box values.
This class represents a basic object for a curve (slur, tie) in the layout domain.
Definition: floatingobject.h:329
void AddGap(int start, int end)
Add a gap in the line.
Definition: boundingbox.h:337
static void CalcThickBezier(const Point bezier[4], int thickness, Point topBezier[4], Point bottomBezier[4])
Calculate the position of the bezier above and below for a thick bezier.
static bool ArePointsClose(const Point &p1, const Point &p2, int margin)
static void ApproximateBezierBoundingBox(const Point bezier[4], Point &pos, int &width, int &height, int &minYPos, int &maxYPos)
Approximate the bounding box of a bezier taking into accound the height and the width.
int GetSegmentCount() const
The number of segments.
Definition: boundingbox.h:361
This class provides resource values.
Definition: resources.h:30
int m_cachedDrawingX
The cached version of the drawingX and drawingY values.
Definition: boundingbox.h:307
static int RectLeftOverlap(const Point rect1[2], const Point rect2[2], int margin, int vMargin)
Calculate the left / right / top / bottom overlap of two rectangle taking into account the margin / v...
This class is an interface for MEI beam elements (beam, beamSpan).
Definition: drawinginterface.h:97
int Intersects(const FloatingCurvePositioner *curve, Accessor type, int margin=0) const
Return intersection between the bounding box and the curve represented by the FloatingPositioner.
This class is used for storing a music font glyph.
Definition: glyph.h:31
std::pair< int, int > GetStartEnd(int idx) const
Get the start and end of a segment.
static double GetBezierThicknessCoefficient(const Point bezier[4], int currentThickness, int penWidth)
Calculate thickness coefficient to be applient for bezier curve to fit MEI units thickness.
This class represents a basic object in the layout domain.
Definition: boundingbox.h:32
Simple class for representing points.
Definition: devicecontextbase.h:203
static Point CalcDeCasteljau(const Point bezier[4], double t)
Calculate the point bezier point position for a t between 0.0 and 1.0.
static Point CalcPositionAfterRotation(Point point, float alpha, Point center)
Calculate the position of a point after a rotation of alpha (in radian) around the center.
void SetBoundingBoxGlyph(char32_t smuflGlyph, int fontSize)
Set and get the smuflGlyph / fontsize for a bounding box that is the one of a single SMuFL glyph.