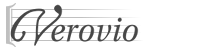 |
Verovio
Source code documentation
|
8 #ifndef __VRV_CALCARTICFUNCTOR_H__
9 #define __VRV_CALCARTICFUNCTOR_H__
41 FunctorCode VisitArtic(
Artic *artic)
override;
42 FunctorCode VisitChord(
Chord *chord)
override;
43 FunctorCode VisitNote(
Note *note)
override;
50 int CalculateHorizontalShift(
const Artic *artic,
bool virtualStem)
const;
60 data_STEMDIRECTION m_stemDir;
68 bool m_crossStaffAbove;
69 bool m_crossStaffBelow;
74 #endif // __VRV_CALCARTICFUNCTOR_H__
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class represents a collection of notes in the same layer with the same onset time.
Definition: chord.h:32
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: calcarticfunctor.h:35
This class models the MEI <note> element.
Definition: note.h:47
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
This class represents a layer in a laid-out score (Doc).
Definition: layer.h:33
This abstract class is the base class for all mutable functors that need access to the document.
Definition: functor.h:151
This class calculates the position of the outside articulations.
Definition: calcarticfunctor.h:22