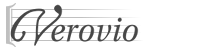 |
Verovio
Source code documentation
|
8 #ifndef __VRV_CALCSTEMFUNCTOR_H__
9 #define __VRV_CALCSTEMFUNCTOR_H__
41 FunctorCode VisitBeam(
Beam *beam)
override;
42 FunctorCode VisitBeamSpan(
BeamSpan *beamSpan)
override;
43 FunctorCode VisitChord(
Chord *chord)
override;
44 FunctorCode VisitFTrem(
FTrem *fTrem)
override;
45 FunctorCode VisitNote(
Note *note)
override;
46 FunctorCode VisitStaff(
Staff *staff)
override;
47 FunctorCode VisitStem(
Stem *stem)
override;
48 FunctorCode VisitTabDurSym(
TabDurSym *tabDurSym)
override;
49 FunctorCode VisitTabGrp(
TabGrp *tabGrp)
override;
59 data_STEMDIRECTION CalcStemDirection(
const Chord *chord,
int verticalCenter)
const;
64 void AdjustFlagPlacement(
65 const Doc *doc,
Stem *stem,
Flag *flag,
int staffSize,
int verticalCenter, data_DURATION duration)
const;
71 int m_chordStemLength;
79 bool m_isStemSameasSecondary;
81 bool m_tabGrpWithNoNote;
92 #endif // __VRV_CALCSTEMFUNCTOR_H__
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class models the MEI <beamSpan> element.
Definition: beamspan.h:31
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class models the MEI <fTrem> element.
Definition: ftrem.h:25
This class models the MEI <tabDurSym> element.
Definition: tabdursym.h:25
This class represents a collection of notes in the same layer with the same onset time.
Definition: chord.h:32
This class models a stem as a layer element part and has no direct MEI equivalent.
Definition: elementpart.h:97
This class models the MEI <tabGrp> element.
Definition: tabgrp.h:23
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: calcstemfunctor.h:35
This class models the MEI <note> element.
Definition: note.h:47
This class is an interface for MEI stemmed element.
Definition: drawinginterface.h:353
This class models a stem as a layer element part and as MEI <stem> element.
Definition: stem.h:27
This class sets the drawing stem positions, including for beams.
Definition: calcstemfunctor.h:22
This class represents a layer in a laid-out score (Doc).
Definition: layer.h:33
This abstract class is the base class for all mutable functors that need access to the document.
Definition: functor.h:151