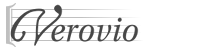 |
Verovio
Source code documentation
|
8 #ifndef __VRV_CASTOFFFUNCTOR_H__
9 #define __VRV_CASTOFFFUNCTOR_H__
41 System *GetLeftoverSystem()
const {
return m_leftoverSystem; }
46 void SetSystemWidth(
int width) { m_systemWidth = width; }
52 FunctorCode VisitDiv(Div *div)
override;
53 FunctorCode VisitEditorialElement(EditorialElement *editorialElement)
override;
54 FunctorCode VisitEnding(Ending *ending)
override;
55 FunctorCode VisitMeasure(Measure *measure)
override;
56 FunctorCode VisitPageElement(PageElement *pageElement)
override;
57 FunctorCode VisitPageMilestone(PageMilestoneEnd *pageMilestoneEnd)
override;
58 FunctorCode VisitSb(Sb *sb)
override;
59 FunctorCode VisitScoreDef(ScoreDef *scoreDef)
override;
60 FunctorCode VisitSystem(System *system)
override;
61 FunctorCode VisitSystemEnd(System *system)
override;
62 FunctorCode VisitSystemElement(SystemElement *systemElement)
override;
63 FunctorCode VisitSystemMilestone(SystemMilestoneEnd *systemMilestoneEnd)
override;
74 System *m_contentSystem;
78 System *m_currentSystem;
84 int m_currentScoreDefWidth;
86 ArrayOfObjects m_pendingElements;
90 System *m_leftoverSystem;
118 void SetLeftoverSystem(
System *system) { m_leftoverSystem = system; }
123 void SetPageHeight(
int height) { m_pageHeight = height; }
129 FunctorCode VisitPageEnd(Page *page)
override;
130 FunctorCode VisitPageElement(PageElement *pageElement)
override;
131 FunctorCode VisitPageMilestone(PageMilestoneEnd *pageMilestoneEnd)
override;
132 FunctorCode VisitScore(Score *score)
override;
133 FunctorCode VisitSystem(System *system)
override;
142 int GetAvailableDrawingHeight()
const;
152 bool m_firstCastOffPage;
162 System *m_leftoverSystem;
164 ArrayOfObjects m_pendingPageElements;
193 FunctorCode VisitDiv(
Div *div)
override;
194 FunctorCode VisitEditorialElement(
EditorialElement *editorialElement)
override;
195 FunctorCode VisitEnding(
Ending *ending)
override;
196 FunctorCode VisitMeasure(
Measure *measure)
override;
197 FunctorCode VisitPageElement(
PageElement *pageElement)
override;
198 FunctorCode VisitPageMilestone(
PageMilestoneEnd *pageMilestoneEnd)
override;
199 FunctorCode VisitPb(
Pb *pb)
override;
200 FunctorCode VisitSb(
Sb *sb)
override;
201 FunctorCode VisitScoreDef(
ScoreDef *scoreDef)
override;
202 FunctorCode VisitStaff(
Staff *staff)
override;
203 FunctorCode VisitSystem(
System *system)
override;
204 FunctorCode VisitSystemElement(
SystemElement *systemElement)
override;
250 void SetResetCache(
bool resetCache) { m_resetCache = resetCache; }
256 FunctorCode VisitFloatingObject(FloatingObject *floatingObject)
override;
257 FunctorCode VisitMeasure(Measure *measure)
override;
258 FunctorCode VisitPageElement(PageElement *pageElement)
override;
259 FunctorCode VisitPageMilestone(PageMilestoneEnd *pageMilestoneEnd)
override;
260 FunctorCode VisitScore(Score *score)
override;
261 FunctorCode VisitSystem(System *system)
override;
274 System *m_currentSystem;
307 FunctorCode VisitDiv(
Div *div)
override;
308 FunctorCode VisitEditorialElement(
EditorialElement *editorialElement)
override;
309 FunctorCode VisitMeasure(
Measure *measure)
override;
310 FunctorCode VisitPageElement(
PageElement *pageElement)
override;
311 FunctorCode VisitPageMilestone(
PageMilestoneEnd *pageMilestoneEnd)
override;
312 FunctorCode VisitScoreDef(
ScoreDef *scoreDef)
override;
313 FunctorCode VisitSystem(
System *system)
override;
314 FunctorCode VisitSystemElement(
SystemElement *systemElement)
override;
341 #endif // __VRV_CASTOFFFUNCTOR_H__
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class represents a measure in a page-based score (Doc).
Definition: measure.h:37
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class represents elements appearing within a measure.
Definition: systemelement.h:25
This class represents an MEI Div.
Definition: div.h:24
This class represents a MEI ending.
Definition: ending.h:28
This class casts off the document according to the encoding provided (pb and sb).
Definition: castofffunctor.h:174
This class represents elements appearing within a page.
Definition: pageelement.h:25
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: castofffunctor.h:113
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: castofffunctor.h:245
This class casts off a document to selection.
Definition: castofffunctor.h:288
This class models an end milestone element at the system level.
Definition: systemmilestone.h:28
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: castofffunctor.h:301
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
This class models an end milestone element and has no MEI equivalent.
Definition: pagemilestone.h:26
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: castofffunctor.h:187
This class undoes the cast off for both pages and systems.
Definition: castofffunctor.h:232
This class represents a MEI pb in score-based MEI.
Definition: pb.h:25
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: castofffunctor.h:36
This class represents a page in a laid-out score (Doc).
Definition: page.h:31
This class represents a MEI sb in score-based MEI.
Definition: sb.h:25
This class represents a system in a laid-out score (Doc).
Definition: system.h:36
This class is a base class for the editorial element containing musical content, for example <rgd> or...
Definition: editorial.h:38
This class represents a MEI scoreDef.
Definition: scoredef.h:129
This class fills a document by adding pages with the appropriate length.
Definition: castofffunctor.h:100
This class fills a page by adding systems with the appropriate length.
Definition: castofffunctor.h:23
This abstract class is the base class for all mutable functors that need access to the document.
Definition: functor.h:151