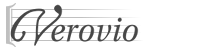 |
Verovio
Source code documentation
|
8 #ifndef __VRV_CONVERTFUNCTOR_H__
9 #define __VRV_CONVERTFUNCTOR_H__
15 #include "alignfunctor.h"
45 FunctorCode VisitDiv(
Div *div)
override;
46 FunctorCode VisitEditorialElement(
EditorialElement *editorialElement)
override;
47 FunctorCode VisitEditorialElementEnd(
EditorialElement *editorialElement)
override;
48 FunctorCode VisitEnding(
Ending *ending)
override;
49 FunctorCode VisitEndingEnd(
Ending *ending)
override;
50 FunctorCode VisitMeasure(
Measure *measure)
override;
51 FunctorCode VisitMdiv(
Mdiv *mdiv)
override;
52 FunctorCode VisitMdivEnd(
Mdiv *mdiv)
override;
53 FunctorCode VisitScore(
Score *score)
override;
54 FunctorCode VisitScoreEnd(
Score *score)
override;
55 FunctorCode VisitScoreDef(
ScoreDef *scoreDef)
override;
56 FunctorCode VisitSection(
Section *section)
override;
57 FunctorCode VisitSectionEnd(
Section *section)
override;
58 FunctorCode VisitSystemElement(
SystemElement *systemElement)
override;
101 FunctorCode VisitLayer(
Layer *layer)
override;
102 FunctorCode VisitMeasure(
Measure *measure)
override;
103 FunctorCode VisitObject(
Object *
object)
override;
104 FunctorCode VisitScoreDef(
ScoreDef *scoreDef)
override;
105 FunctorCode VisitStaff(
Staff *staff)
override;
106 FunctorCode VisitSystemElement(
SystemElement *systemElement)
override;
113 bool IsValidBreakPoint(
const Alignment *alignment,
const int nbLayers);
115 void InitSegment(
Object *
object);
121 std::list<Measure *> m_segments;
123 std::list<Measure *>::iterator m_currentSegment;
125 std::list<const Alignment *> m_breakPoints;
127 std::list<const Alignment *>::const_iterator m_currentBreakPoint;
129 Staff *m_contentStaff;
130 Layer *m_contentLayer;
133 Staff *m_targetStaff;
134 Layer *m_targetLayer;
164 void TrackSegmentsToDelete(
bool trackSegments) { m_trackSegmentsToDelete = trackSegments; }
165 const ArrayOfObjects &GetSegmentsToDelete()
const {
return m_segmentsToDelete; }
172 FunctorCode VisitLayer(Layer *layer)
override;
173 FunctorCode VisitMeasure(Measure *measure)
override;
174 FunctorCode VisitSection(Section *section)
override;
185 Measure *m_contentMeasure;
186 Layer *m_contentLayer;
188 bool m_trackSegmentsToDelete;
190 ArrayOfObjects m_segmentsToDelete;
220 FunctorCode VisitChord(
Chord *chord)
override;
221 FunctorCode VisitLayer(
Layer *layer)
override;
222 FunctorCode VisitLayerElement(
LayerElement *
object)
override;
223 FunctorCode VisitLigature(
Ligature *ligature)
override;
224 FunctorCode VisitLigatureEnd(
Ligature *ligature)
override;
225 FunctorCode VisitMeasure(
Measure *measure)
override;
226 FunctorCode VisitMeasureEnd(
Measure *measure)
override;
227 FunctorCode VisitNote(
Note *note)
override;
228 FunctorCode VisitRest(
Rest *rest)
override;
229 FunctorCode VisitScoreDef(
ScoreDef *scoreDef)
override;
230 FunctorCode VisitStaff(
Staff *staff)
override;
231 FunctorCode VisitStaffEnd(
Staff *staff)
override;
232 FunctorCode VisitSystemElement(
SystemElement *systemElement)
override;
233 FunctorCode VisitSystemEnd(
System *system)
override;
240 bool IsGlobalMensur(
const Alignment *alignment,
const int nbLayers,
Mensur &mensur);
246 void SplitDurationInterface(ClassId classId, data_DURATION elementDur,
Fraction time,
Fraction duration);
248 void ConvertAccid(
Note *cmnNote,
const Accid *accid,
bool &isFirstNote);
250 void ConvertClef(
Clef *cmnClef,
const Clef *clef);
252 void ConvertMensur(
const Mensur *mensur);
264 MeasureInfo(
const Fraction &time,
const Fraction &duration) : m_time(time), m_duration(duration)
278 CmnDuration(data_DURATION duration,
int dots,
int num = 1,
int numbase = 1)
279 : m_duration(duration), m_dots(dots), m_num(num), m_numbase(numbase)
284 data_DURATION m_duration;
293 void SplitDurationIntoCmn(
294 data_DURATION elementDur, Fraction duration,
const Mensur *mensur, std::list<CmnDuration> &cmnDurations);
302 std::vector<MeasureInfo> m_measures;
304 std::vector<MeasureInfo>::iterator m_currentMeasure;
306 std::vector<Layer *> m_layers;
308 std::vector<Layer *>::iterator m_currentLayer;
310 std::list<Clef *> m_clefs;
314 System *m_targetSystem;
316 AlignMeterParams m_currentParams;
318 ListOfObjects m_durationElements;
320 BracketSpan *m_ligature;
322 BracketSpan *m_coloration;
324 Tuplet *m_proportTuplet;
326 Staff *m_currentStaff;
328 std::string m_startid;
358 const std::vector<Note *> &GetCurrentNotes()
const {
return m_currentNotes; }
364 FunctorCode VisitChord(Chord *chord)
override;
365 FunctorCode VisitChordEnd(Chord *chord)
override;
366 FunctorCode VisitMeasureEnd(Measure *measure)
override;
367 FunctorCode VisitMRest(MRest *mRest)
override;
368 FunctorCode VisitNote(Note *note)
override;
369 FunctorCode VisitRest(Rest *rest)
override;
376 void ConvertToFermata(Fermata *fermata, AttFermataPresent *fermataPresent,
const std::string &
id);
382 std::vector<Note *> m_currentNotes;
384 Chord *m_currentChord;
386 ArrayOfObjects m_controlEvents;
417 FunctorCode VisitArtic(
Artic *artic)
override;
418 FunctorCode VisitLayerEnd(
Layer *layer)
override;
427 void SplitMultival(
Artic *artic)
const;
433 std::vector<Artic *> m_articsToConvert;
463 FunctorCode VisitScore(
Score *score)
override;
464 FunctorCode VisitScoreDefElement(
ScoreDefElement *scoreDefElement)
override;
465 FunctorCode VisitScoreDefElementEnd(
ScoreDefElement *scoreDefElement)
override;
506 FunctorCode VisitEditorialElement(
EditorialElement *editorialElement)
override;
507 FunctorCode VisitLayer(
Layer *layer)
override;
508 FunctorCode VisitLayerEnd(
Layer *layer)
override;
509 FunctorCode VisitLayerElement(
LayerElement *layerElement)
override;
510 FunctorCode VisitLayerElementEnd(
LayerElement *layerElement)
override;
511 FunctorCode VisitLigature(
Ligature *ligature)
override;
512 FunctorCode VisitLigatureEnd(
Ligature *ligature)
override;
525 ListOfObjects m_stack;
530 #endif // __VRV_CONVERTFUNCTOR_H__
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class represents a measure in a page-based score (Doc).
Definition: measure.h:37
This class converts markup of artic@artic multi value into distinct artic elements.
Definition: convertfunctor.h:398
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class represents elements appearing within a measure.
Definition: systemelement.h:25
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:411
This class represents an MEI Div.
Definition: div.h:24
This class represents a MEI ending.
Definition: ending.h:28
This class converts cast-off (measure) mensural segments MEI into mensural.
Definition: convertfunctor.h:144
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:353
This class represents a MEI section.
Definition: section.h:28
This class represents a collection of notes in the same layer with the same onset time.
Definition: chord.h:32
This class models the MEI <accid> element.
Definition: accid.h:27
This class is a base class for MEI scoreDef or staffDef elements.
Definition: scoredef.h:42
This class represents a basic object.
Definition: object.h:59
This class converts all top-level containers (section, endings) and editorial elements to milestone e...
Definition: convertfunctor.h:26
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:457
This class converts mensural MEI into cast-off (measure) segments looking at the barLine objects.
Definition: convertfunctor.h:201
This class represents a collection of notes in the same layer with successive onset times,...
Definition: ligature.h:28
Definition: fraction.h:19
This class models the MEI <mensur> element.
Definition: mensur.h:27
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:39
This class converts mensural MEI into cast-off (measure) segments looking at the barLine objects.
Definition: convertfunctor.h:82
This class moves scoreDef clef, keySig, meterSig and mensur to staffDef.
Definition: convertfunctor.h:487
This class models the MEI <clef> element.
Definition: clef.h:27
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:157
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:500
This class stores an alignment position elements will point to.
Definition: horizontalaligner.h:73
This class converts analytical markup (@fermata, @tie) to elements.
Definition: convertfunctor.h:340
This class models the MEI <note> element.
Definition: note.h:47
This class represent a <mdiv> in page-based MEI.
Definition: mdiv.h:24
This class represents a page in a laid-out score (Doc).
Definition: page.h:31
This class represents a system in a laid-out score (Doc).
Definition: system.h:36
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:214
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: convertfunctor.h:95
This class is a base class for the editorial element containing musical content, for example <rgd> or...
Definition: editorial.h:38
This class represents a MEI scoreDef.
Definition: scoredef.h:129
This class models the MEI <rest> element.
Definition: rest.h:37
This class is an interface for elements with duration, such as notes and rests.
Definition: durationinterface.h:31
This class represent a <score> in MEI.
Definition: score.h:30
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
This class represents a layer in a laid-out score (Doc).
Definition: layer.h:33
This abstract class is the base class for all mutable functors that need access to the document.
Definition: functor.h:151
This class moves scoreDef clef, keySig, meterSig and mensur to staffDef.
Definition: convertfunctor.h:444