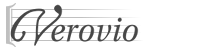 |
Verovio
Source code documentation
|
11 #include "devicecontextbase.h"
12 #include "expansionmap.h"
13 #include "facsimile.h"
15 #include "resources.h"
32 enum DocType { Raw = 0, Rendering, Transcription, Facs };
61 void Reset()
override;
66 void ResetToLoading();
79 void SetOptions(
Options *options) { (*m_options) = *options; }
87 Resources &GetResourcesForModification() {
return m_resources; }
124 DocType
GetType()
const {
return m_type; }
126 void SetType(DocType type);
127 bool IsFacs()
const {
return (m_type == Facs); }
128 bool IsRaw()
const {
return (m_type == Raw); }
129 bool IsRendering()
const {
return (m_type == Rendering); }
130 bool IsTranscription()
const {
return (m_type == Transcription); }
136 bool HasPage(
int pageIdx)
const;
167 Score *GetFirstVisibleScore();
189 int GetGlyphHeight(char32_t code,
int staffSize,
bool graceSize)
const;
191 int GetGlyphWidth(char32_t code,
int staffSize,
bool graceSize)
const;
192 int GetGlyphLeft(char32_t code,
int staffSize,
bool graceSize)
const;
193 int GetGlyphRight(char32_t code,
int staffSize,
bool graceSize)
const;
194 int GetGlyphBottom(char32_t code,
int staffSize,
bool graceSize)
const;
195 int GetGlyphTop(char32_t code,
int staffSize,
bool graceSize)
const;
196 int GetGlyphAdvX(char32_t code,
int staffSize,
bool graceSize)
const;
197 int GetDrawingUnit(
int staffSize)
const;
198 int GetDrawingDoubleUnit(
int staffSize)
const;
199 int GetDrawingStaffSize(
int staffSize)
const;
200 int GetDrawingOctaveSize(
int staffSize)
const;
201 int GetDrawingBrevisWidth(
int staffSize)
const;
202 int GetDrawingBarLineWidth(
int staffSize)
const;
203 int GetDrawingStaffLineWidth(
int staffSize)
const;
204 int GetDrawingStemWidth(
int staffSize)
const;
205 int GetDrawingDirHeight(
int staffSize,
bool withMargin)
const;
206 int GetDrawingDynamHeight(
int staffSize,
bool withMargin)
const;
207 int GetDrawingHairpinSize(
int staffSize,
bool withMargin)
const;
208 int GetDrawingBeamWidth(
int staffSize,
bool graceSize)
const;
209 int GetDrawingBeamWhiteWidth(
int staffSize,
bool graceSize)
const;
210 int GetDrawingLedgerLineExtension(
int staffSize,
bool graceSize)
const;
211 int GetDrawingMinimalLedgerLineExtension(
int staffSize,
bool graceSize)
const;
212 int GetCueSize(
int value)
const;
213 double GetCueScaling()
const;
216 Point ConvertFontPoint(
const Glyph *glyph,
const Point &fontPoint,
int staffSize,
bool graceSize)
const;
222 int GetTextGlyphHeight(char32_t code,
const FontInfo *font,
bool graceSize)
const;
224 int GetTextGlyphWidth(char32_t code,
const FontInfo *font,
bool graceSize)
const;
225 int GetTextGlyphAdvX(char32_t code,
const FontInfo *font,
bool graceSize)
const;
226 int GetTextGlyphDescender(char32_t code,
const FontInfo *font,
bool graceSize)
const;
227 int GetTextLineHeight(
const FontInfo *font,
bool graceSize)
const;
228 int GetTextXHeight(
const FontInfo *font,
bool graceSize)
const;
235 FontInfo *GetDrawingSmuflFont(
int staffSize,
bool graceSize);
237 FontInfo *GetDrawingLyricFont(
int staffSize);
238 FontInfo *GetFingeringFont(
int staffSize);
251 double GetLeftMargin(
const ClassId classId)
const;
253 double GetLeftMargin(
const Object *
object)
const;
254 double GetRightMargin(
const ClassId classId)
const;
255 double GetRightMargin(
const Object *
object)
const;
256 double GetLeftPosition()
const;
257 double GetBottomMargin(
const ClassId classId)
const;
258 double GetTopMargin(
const ClassId classId)
const;
265 data_MEASUREMENTSIGNED
GetStaffDistance(
const Object *
object,
int staffIndex, data_STAFFREL staffPosition)
const;
290 bool ExportTimemap(std::string &output,
bool includeRests,
bool includeMeasures,
bool useFractions);
300 bool ExportFeatures(std::string &output,
const std::string &options);
399 void ConvertToMensuralViewDoc();
486 void SetMensuralMusicOnly(data_BOOLEAN isMensuralMusicOnly);
488 bool IsMensuralMusicOnly()
const {
return (m_isMensuralMusicOnly == BOOLEAN_true); }
494 void SetNeumeLines(
bool isNeumeLines) { m_isNeumeLines = isNeumeLines; }
496 bool IsNeumeLines()
const {
return m_isNeumeLines; }
502 void SetFacsimile(Facsimile *facsimile) { m_facsimile = facsimile; }
504 Facsimile *GetFacsimile() {
return m_facsimile; }
505 const Facsimile *GetFacsimile()
const {
return m_facsimile; }
506 bool HasFacsimile()
const {
return m_facsimile != NULL; }
517 void InitSelectionDoc(
DocSelection &selection,
bool resetCache);
519 void ResetSelectionDoc(
bool resetCache);
520 bool HasSelection()
const;
527 void ReactivateSelection(
bool resetAligners);
550 FunctorCode AcceptEnd(
Functor &functor)
override;
551 FunctorCode AcceptEnd(
ConstFunctor &functor)
const override;
558 int CalcMusicFontSize();
563 void PrepareMeasureIndices();
568 void CollectVisibleScores();
576 Page *m_selectionPreceding;
577 Page *m_selectionFollowing;
578 std::string m_selectionStart;
579 std::string m_selectionEnd;
657 std::list<Score *> m_visibleScores;
667 FocusStatusType m_focusStatus;
681 int m_drawingBeamWidth;
683 int m_drawingBeamWhiteWidth;
685 int m_drawingBrevisWidth;
688 int m_drawingSmuflFontSize;
690 int m_drawingLyricFontSize;
692 int m_fingeringFontSize;
705 bool m_currentScoreDefDone;
711 bool m_dataPreparationDone;
718 double m_timemapTempo;
731 data_BOOLEAN m_isMensuralMusicOnly;
744 int m_pageMarginBottom;
746 int m_pageMarginLeft;
748 int m_pageMarginRight;
bool IsSupportedChild(ClassId classId) override
Add a page to the document.
void ConvertMensuralToCmnDoc()
Convert the doc from mensural to CMN.
void CastOffEncodingDoc()
Cast off of the entire document according to the encoded data (pb and sb).
pugi::xml_document m_front
A copy of the front tree stored as pugi::xml_document.
Definition: doc.h:594
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
void ExportMIDI(smf::MidiFile *midiFile)
Export the document to a MIDI file.
bool HasTimemap() const
Check to see if the timemap has already been calculated.
int GetAdjustedDrawingPageWidth() const
Return the width adjusted to the content of the current drawing page.
void GenerateMEIHeader()
Generate a minimal MEI header.
bool GenerateMeasureNumbers()
Generate measure numbers from measure attributes.
bool GenerateDocumentScoreDef()
Generate a document scoreDef when none is provided.
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
int m_drawingPageContentHeight
The current page content height (without margings)
Definition: doc.h:611
int m_drawingPageMarginBottom
The current page bottom margin.
Definition: doc.h:615
void SetFocus()
Reset the document focus.
pugi::xml_document m_header
A copy of the header tree stored as pugi::xml_document.
Definition: doc.h:589
int m_drawingPageMarginRight
The current page right margin.
Definition: doc.h:619
void UnCastOffDoc(bool resetCache=true)
Undo the cast off of the entire document.
void RefreshLayout()
Refresh the layout of all pages in the doc.
bool GetMidiExportDone() const
Return true if the MIDI generation is already done.
bool IsCastOff() const
Return true if the document has been cast off already.
Definition: doc.h:512
std::list< Score * > GetVisibleScores()
Get all visible scores / the first visible score Lazily updates the visible scores,...
This class represents a basic object.
Definition: object.h:59
This class represent a <pages> in page-based MEI.
Definition: pages.h:27
float m_drawingBeamMaxSlope
the current beam maximal slope
Definition: doc.h:623
Pages * GetPages()
Get the Pages in the visible Mdiv.
pugi::xml_document m_back
A copy of the back tree stored as pugi::xml_document.
Definition: doc.h:599
void ScoreDefOptimizeDoc()
Optimize the scoreDef once the document is cast-off.
bool ExportFeatures(std::string &output, const std::string &options)
Extract music features to JSON string.
int m_drawingPageHeight
The current page height.
Definition: doc.h:607
void CastOffSmartDoc()
Casts off the entire document, only using the document's system breaks if they would be close to the ...
void ConvertToCastOffMensuralDoc(bool castOff)
Convert mensural MEI into cast-off (measure) segments looking at the barLine objects.
bool CheckPageSize(const Page *page) const
Check that the page is the drawing page or that they have no given dimensions.
std::string m_musicDecls
The music@decls value.
Definition: doc.h:604
This class is store font properties.
Definition: devicecontextbase.h:137
This class represent a page range not owning child pages.
Definition: pages.h:87
int GetAdjustedDrawingPageHeight() const
Return the height adjusted to the content of the current drawing page.
ExpansionMap m_expansionMap
An expansion map that contains
Definition: doc.h:632
This class stores a document selection.
Definition: docselection.h:24
void ScoreDefSetGrpSymDoc()
Set the GrpSym start / end for each System once ScoreDef is set and (if necessary) optimized.
void UpdatePageDrawingSizes()
Update the drawing page sizes when a page is set as drawing page.
void CastOffDocBase(bool useSb, bool usePb, bool smart=false)
Casts off the entire document, with options for obeying breaks.
DocType GetType() const
Getter and setter for the DocType.
Definition: doc.h:125
void ScoringUpDoc()
Calls the scoringUpFunctor and applies it.
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
bool HasPage(int pageIdx) const
Check if the document has a page with the specified value.
void SyncToFacsimileDoc()
Sync the coordinate provided in rendering to a <facsimile>.
void ExpandExpansions()
Convert encoded <expansion> before rendering.
This class provides resource values.
Definition: resources.h:30
Options * GetOptions()
Getter for the options.
Definition: doc.h:77
void SyncFromFacsimileDoc()
Sync the coordinate provided trought <facsimile> to m_drawingFacsX/Y.
void Reset() override
Clear the content of the document.
This class represents a page in a laid-out score (Doc).
Definition: page.h:31
This abstract class is the base class for all const functors.
Definition: functor.h:126
PageRange * m_focusRange
A page range (owned object) with focus in the document.
Definition: doc.h:584
Implements the facsimile element in MEI.
Definition: facsimile.h:31
int m_drawingPageWidth
The current page width.
Definition: doc.h:609
double GetMusicToLyricFontSizeRatio() const
Get the ratio between the lyric font size and the music font size.
ScoreDef * GetFirstScoreDef()
Get the first scoreDef.
int m_drawingPageContentWidth
The current page content width (without margins)
Definition: doc.h:613
void GenerateFooter()
Generate a document pgFoot if none is provided.
void ClearSelectionPages()
Clear the selection pages.
bool ExportTimemap(std::string &output, bool includeRests, bool includeMeasures, bool useFractions)
Extract a timemap from the document to a JSON string.
data_MEASUREMENTSIGNED GetStaffDistance(const Object *object, int staffIndex, data_STAFFREL staffPosition) const
Get the default distance from the staff for the object The distance is given in x * MEI UNIT.
void PrepareData()
Prepare the document data.
bool ExportExpansionMap(std::string &output)
Extract expansionMap from the document to JSON string.
This class is used for storing a music font glyph.
Definition: glyph.h:31
Score * GetCorrespondingScore(const Object *object)
Get the corresponding score for a node.
void ConvertToPageBasedDoc()
Convert the doc from score-based to page-based MEI.
Page * GetDrawingPage()
Getter to the drawPage.
Definition: doc.h:455
int GetPageCount() const
Get the total page count.
void TransposeDoc()
Transpose the content of the doc.
void CastOffDoc()
Casts off the entire document.
This class represents a MEI scoreDef.
Definition: scoredef.h:129
void ResetDataPage()
Reset drawing page to NULL.
Definition: doc.h:447
void ConvertHeaderToMEIBasic()
Convert the header to MEI basic by preserving only the fileDesc and its titleStmt.
const Resources & GetResources() const
Getter for the resources.
Definition: doc.h:86
This class represent a <score> in MEI.
Definition: score.h:30
void ScoreDefSetCurrentDoc(bool force=false)
Set the initial scoreDef of each page.
int m_drawingPageMarginTop
The current page top margin.
Definition: doc.h:621
data_NOTATIONTYPE m_notationType
Record notation type for document.
Definition: doc.h:629
Definition: expansionmap.h:19
Simple class for representing points.
Definition: devicecontextbase.h:203
void SetMarkup(int markup)
Setter for markup flag.
Definition: doc.h:481
void CalculateTimemap()
Prepare the timemap for MIDI and timemap file export.
void ConvertToCmnDoc()
Convert mensural MEI into CMN measure-based MEI.
This class contains the document styling parameters.
Definition: options.h:576
void CastOffLineDoc()
Casts off the entire document, using the document's line breaks, but adding its own page breaks.
void DeactiveateSelection()
Temporarily deactivate and reactivate selection.
Page * SetDrawingPage(int pageIdx, bool withPageRange=false)
Set drawing values (page size, etc) when drawing a page.
int m_drawingPageMarginLeft
The current page left margin.
Definition: doc.h:617
void ConvertMarkupDoc(bool permanent=true)
Convert analytical encoding (@fermata, @tie) to correpsonding elements By default,...
void GenerateHeader()
Generate a document pgHead from the MEI header if none is provided.