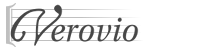 |
Verovio
Source code documentation
|
8 #ifndef __VRV_DRAWING_INTERFACE_H__
9 #define __VRV_DRAWING_INTERFACE_H__
12 #include "devicecontextbase.h"
16 #include "metersiggrp.h"
105 virtual void Reset();
121 void InitCoords(
const ArrayOfObjects &childList,
Staff *staff, data_BEAMPLACE place);
122 void InitCoords(
const ListOfObjects &childList,
Staff *staff, data_BEAMPLACE place);
137 bool IsHorizontal()
const;
139 bool IsRepeatedPattern()
const;
201 bool IsHorizontalMixedBeam(
const std::vector<int> &items,
const std::vector<data_BEAMPLACE> &directions)
const;
207 bool m_hasMultipleStemDir;
209 Staff *m_crossStaffContent;
210 data_STAFFREL_basic m_crossStaffRel;
211 bool m_isSpanningElement;
212 data_DURATION m_shortestDur;
213 data_STEMDIRECTION m_notesStemDir;
214 data_BEAMPLACE m_drawingPlace;
219 int m_beamWidthBlack;
220 int m_beamWidthWhite;
252 virtual void Reset();
260 bool DrawClef()
const {
return (m_drawClef && m_currentClef.HasShape()); }
262 void SetDrawClef(
bool drawClef) { m_drawClef = drawClef; }
263 bool DrawKeySig()
const {
return (m_drawKeySig); }
264 void SetDrawKeySig(
bool drawKeySig) { m_drawKeySig = drawKeySig; }
265 bool DrawMensur()
const {
return (m_drawMensur && (m_currentMensur.HasSign() || m_currentMensur.HasNum())); }
266 void SetDrawMensur(
bool drawMensur) { m_drawMensur = drawMensur; }
267 bool DrawMeterSig()
const
269 return (m_drawMeterSig && (m_currentMeterSig.HasUnit() || m_currentMeterSig.HasSym()));
271 void SetDrawMeterSig(
bool drawMeterSig) { m_drawMeterSig = drawMeterSig; }
272 bool DrawMeterSigGrp()
const;
273 void SetDrawMeterSigGrp(
bool drawMeterSigGrp) { m_drawMeterSigGrp = drawMeterSigGrp; }
279 void SetCurrentClef(
const Clef *clef);
281 void SetCurrentKeySig(
const KeySig *keySig);
282 void SetCurrentMensur(
const Mensur *mensur);
283 void SetCurrentMeterSig(
const MeterSig *meterSig);
284 void SetCurrentMeterSigGrp(
const MeterSigGrp *meterSigGrp);
285 void AlternateCurrentMeterSig(
const Measure *measure);
286 void SetCurrentProport(
const Proport *proport);
293 Clef *GetCurrentClef() {
return &m_currentClef; }
295 const Clef *GetCurrentClef()
const {
return &m_currentClef; }
296 KeySig *GetCurrentKeySig() {
return &m_currentKeySig; }
297 const KeySig *GetCurrentKeySig()
const {
return &m_currentKeySig; }
298 Mensur *GetCurrentMensur() {
return &m_currentMensur; }
299 const Mensur *GetCurrentMensur()
const {
return &m_currentMensur; }
300 MeterSig *GetCurrentMeterSig() {
return &m_currentMeterSig; }
301 const MeterSig *GetCurrentMeterSig()
const {
return &m_currentMeterSig; }
302 MeterSigGrp *GetCurrentMeterSigGrp() {
return &m_currentMeterSigGrp; }
303 const MeterSigGrp *GetCurrentMeterSigGrp()
const {
return &m_currentMeterSigGrp; }
304 Proport *GetCurrentProport() {
return &m_currentProport; }
305 const Proport *GetCurrentProport()
const {
return &m_currentProport; }
341 bool m_drawMeterSigGrp;
361 virtual void Reset();
367 void SetDrawingStem(
Stem *stem);
377 void SetDrawingStemDir(data_STEMDIRECTION stemDir);
379 data_STEMDIRECTION GetDrawingStemDir()
const;
380 void SetDrawingStemLen(
int drawingStemLen);
381 int GetDrawingStemLen()
const;
382 int GetDrawingStemModRelY()
const;
385 Point GetDrawingStemStart(
const Object *
object = NULL)
const;
386 Point GetDrawingStemEnd(
const Object *
object = NULL)
const;
391 virtual Point GetStemUpSE(
const Doc *doc,
int staffSize,
bool graceSize)
const = 0;
393 virtual Point GetStemDownNW(
const Doc *doc,
int staffSize,
bool graceSize)
const = 0;
394 virtual int CalcStemLenInThirdUnits(
const Staff *staff, data_STEMDIRECTION stemDir)
const = 0;
bool HasOneStepHeight() const
Checks whether difference between highest and lowest notes of the beam is just one step.
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class represents a measure in a page-based score (Doc).
Definition: measure.h:37
int GetPosition(const LayerElement *element) const
Return the position of the element in the beam.
virtual std::pair< int, int > GetAdditionalBeamCount() const
Helper to find number of additional beams.
Definition: drawinginterface.h:160
void ClearCoords()
Clear the m_beamElementCoords vector and delete all the objects.
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class models the MEI <meterSig> element.
Definition: metersig.h:27
ArrayOfObjects * GetDrawingList()
Return the drawing list.
This class is an interface for elements with duration, such as notes and rests.
Definition: drawinginterface.h:33
This class represents a basic object.
Definition: object.h:59
ArrayOfBeamElementCoords m_beamElementCoords
An array of coordinates for each element.
Definition: drawinginterface.h:226
void InitCue(bool beamCue)
Initialize m_cueSize value based on the @cue attribute and presence of child elements with @cue attri...
void GetBeamChildOverflow(StaffAlignment *&above, StaffAlignment *&below) const
Get above/below overflow for the children.
This class represents a MEI meterSigGrp.
Definition: metersiggrp.h:28
FunctorCode InterfaceResetData(ResetDataFunctor &functor)
Called explicitly from ResetDataFunctor.
This class models the MEI <mensur> element.
Definition: mensur.h:27
int GetTotalBeamWidth() const
Get total beam width with regards to the shortest duration of the beam (counting both beams and white...
virtual std::pair< int, int > GetFloatingBeamCount() const
Helper to get number of beams represented by attributes @beam and @beam.float.
Definition: drawinginterface.h:165
Stem * m_drawingStem
A pointer to the child Stem set by PrepareLayerElementParts.
Definition: drawinginterface.h:412
bool HasCoords() const
Initializes the m_beamElementCoords vector objects.
Definition: drawinginterface.h:120
This class models the MEI <clef> element.
Definition: clef.h:27
bool IsFirstIn(const LayerElement *element) const
Return information about the position in the beam.
This class is an interface for MEI scoreDef or staffDef attributes clef, keysig and mensur.
Definition: drawinginterface.h:244
void GetBeamOverflow(StaffAlignment *&above, StaffAlignment *&below) const
Get above/below overflow.
This class models the MEI <proport> element.
Definition: proport.h:23
void ResetDrawingList()
Reset the drawing list.
This class is an pseudo interface for elements maintaining a flat list of children LayerElement for p...
Definition: object.h:873
FunctorCode InterfaceResetData(ResetDataFunctor &functor)
Called explicitly from ResetDataFunctor.
FunctorCode InterfaceResetData(ResetDataFunctor &functor)
Called explicitly from ResetDataFunctor.
This class is an interface for MEI beam elements (beam, beamSpan).
Definition: drawinginterface.h:97
ArrayOfObjects m_drawingList
The list of object for which drawing is postponed.
Definition: drawinginterface.h:82
This class is an interface for MEI stemmed element.
Definition: drawinginterface.h:353
void AddToDrawingList(Object *element)
Add an element to the drawing list.
This class models a stem as a layer element part and as MEI <stem> element.
Definition: stem.h:27
FunctorCode InterfaceResetData(ResetDataFunctor &functor)
Called explicitly from ResetDataFunctor.
This class models the MEI <keySig> element.
Definition: keysig.h:44
This class stores an alignement position staves will point to.
Definition: verticalaligner.h:172
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
Simple class for representing points.
Definition: devicecontextbase.h:203
This class resets the drawing values before calling PrepareData after changes.
Definition: resetfunctor.h:22
void InitGraceStemDir(bool graceGrp)
Initialize m_notesStemDir value based on the @graceGrp attribute and presence of child elements with ...