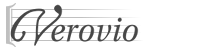 |
Verovio
Source code documentation
|
8 #ifndef __VRV_FINDFUNCTOR_H__
9 #define __VRV_FINDFUNCTOR_H__
43 void SetContinueDepthSearchForMatches(
bool continueDepthSearchForMatches);
49 FunctorCode VisitObject(
Object *
object)
override;
62 bool m_continueDepthSearchForMatches;
64 ListOfObjects *m_elements;
92 void SetContinueDepthSearchForMatches(
bool continueDepthSearchForMatches);
98 FunctorCode VisitObject(
const Object *
object)
override;
111 bool m_continueDepthSearchForMatches;
113 ListOfConstObjects *m_elements;
142 FunctorCode VisitObject(
const Object *
object)
override;
155 const Object *m_start, *m_end;
157 ListOfConstObjects *m_elements;
185 const Object *GetElement()
const {
return m_element; }
191 FunctorCode VisitObject(
const Object *
object)
override;
202 Comparison *m_comparison;
204 const Object *m_element;
232 const Object *GetElement()
const {
return m_element; }
238 FunctorCode VisitObject(
const Object *
object)
override;
251 const Object *m_element;
279 const Object *GetElement()
const {
return m_element; }
285 FunctorCode VisitObject(
const Object *
object)
override;
296 Comparison *m_comparison;
298 const Object *m_start;
300 const Object *m_element;
328 const Object *GetElement()
const {
return m_element; }
334 FunctorCode VisitObject(
const Object *
object)
override;
345 Comparison *m_comparison;
347 const Object *m_start;
349 const Object *m_element;
377 const Object *GetElement()
const {
return m_element; }
383 FunctorCode VisitObject(
const Object *
object)
override;
394 Comparison *m_comparison;
396 const Object *m_element;
425 void IncludeMilestoneReferences(
bool included) { m_milestoneReferences = included; }
431 FunctorCode VisitObject(Object *
object)
override;
438 void AddObject(Object *
object,
const std::string &attribute);
444 SetOfObjects *m_elements;
446 ListOfObjectAttNamePairs *m_listWithAttName;
448 bool m_milestoneReferences;
477 FunctorCode VisitObject(
Object *
object)
override;
490 ListOfObjectAttNamePairs *m_elements;
518 const Object *GetElement()
const {
return m_element; }
524 FunctorCode VisitLayer(
const Layer *layer)
override;
525 FunctorCode VisitScore(
const Score *score)
override;
536 const Object *m_element;
567 FunctorCode VisitObject(
const Object *
object)
override;
578 ListOfConstObjects *m_flatList;
583 #endif // __VRV_FINDFUNCTOR_H__
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:372
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:420
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:513
This class finds all elements in the tree by comparison between start and end objects.
Definition: findfunctor.h:123
This class looks for an element with given ID in StaffDef elements (Clef, KeySig, etc....
Definition: findfunctor.h:500
This class represents a basic object.
Definition: object.h:59
This class finds the last object matching the comparison.
Definition: findfunctor.h:359
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:180
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:274
This class finds all elements in the tree by comparison.
Definition: findfunctor.h:74
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:38
This class finds all objects to which another object refers to.
Definition: findfunctor.h:407
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
This class finds the next child matching the comparison object.
Definition: findfunctor.h:261
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:136
This class adds elements and its children to a flat list.
Definition: findfunctor.h:548
This abstract class is the base class for all const functors.
Definition: functor.h:126
This class finds an element in the tree by comparison.
Definition: findfunctor.h:167
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:87
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:227
This class finds the previous child matching the comparison object.
Definition: findfunctor.h:310
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:471
This class finds all objects referring to a specific object.
Definition: findfunctor.h:458
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:323
This class finds an element with a specified id.
Definition: findfunctor.h:214
Definition: comparison.h:31
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: findfunctor.h:561
This class finds all elements in the tree by comparison.
Definition: findfunctor.h:25