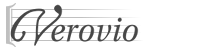 |
Verovio
Source code documentation
|
8 #ifndef __VRV_FLOATING_OBJECT_H__
9 #define __VRV_FLOATING_OBJECT_H__
11 #include "devicecontextbase.h"
17 class FloatingPositioner;
38 void Reset()
override;
42 void UpdateContentBBoxX(
int x1,
int x2)
override;
43 void UpdateContentBBoxY(
int y1,
int y2)
override;
44 void UpdateSelfBBoxX(
int x1,
int x2)
override;
45 void UpdateSelfBBoxY(
int y1,
int y2)
override;
50 int GetDrawingX()
const override;
52 int GetDrawingY()
const override;
61 const FloatingPositioner *GetCurrentFloatingPositioner()
const {
return m_currentPositioner; }
77 int GetDrawingGrpId()
const {
return m_drawingGrpId; }
79 void SetDrawingGrpId(
int drawingGrpId) { m_drawingGrpId = drawingGrpId; }
80 int SetDrawingGrpObject(
void *drawingGrpObject);
87 void ResetMaxDrawingYRel() { m_maxDrawingYRel = VRV_UNSET; }
89 void SetMaxDrawingYRel(
int maxDrawingYRel, data_STAFFREL place);
90 int GetMaxDrawingYRel()
const {
return m_maxDrawingYRel; };
96 void ResetDrawingObjectIDs();
117 const BoundingBox *horizOverlappingBBox,
bool contentTop)
const;
129 FunctorCode AcceptEnd(
Functor &functor)
override;
130 FunctorCode AcceptEnd(
ConstFunctor &functor)
const override;
143 int m_maxDrawingYRel;
155 static thread_local std::vector<void *> s_drawingObjectIds;
170 ClassId GetClassId()
const override {
return FLOATING_POSITIONER; }
172 virtual void ResetPositioner();
177 int GetDrawingX()
const override;
179 int GetDrawingY()
const override;
182 void ResetCachedDrawingX()
const override;
183 void ResetCachedDrawingY()
const override;
190 Object *GetObjectX() {
return m_objectX; }
191 const Object *GetObjectX()
const {
return m_objectX; }
192 Object *GetObjectY() {
return m_objectY; }
193 const Object *GetObjectY()
const {
return m_objectY; }
217 data_STAFFREL GetDrawingPlace()
const {
return m_place; }
225 virtual void SetDrawingYRel(
int drawingYRel,
bool force =
false);
227 virtual void SetDrawingXRel(
int drawingXRel);
253 void CalcDrawingYRel(
const Doc *doc,
const StaffAlignment *staffAlignment,
const BoundingBox *horizOverlappingBBox);
264 const Doc *doc,
const StaffAlignment *staffAlignment,
const BoundingBox *horizOverlappingBBox)
const;
272 const Doc *doc,
const BoundingBox *horizOverlappingBBox,
bool contentTop)
const;
273 int GetVerticalContentBoundary(
const Doc *doc,
const BoundingBox *horizOverlappingBBox,
bool contentTop)
const;
313 data_STAFFREL m_place;
334 ClassId GetClassId()
const override {
return FLOATING_CURVE_POSITIONER; }
336 void ResetPositioner()
override;
358 void MoveBackHorizontal(
int distance);
359 void MoveFrontVertical(
int distance);
360 void MoveBackVertical(
int distance);
374 const BoundingBox *boundingBox,
bool &discard,
int margin = 0,
bool horizontalOverlap =
true)
const;
375 int CalcDirectionalAdjustment(
const BoundingBox *boundingBox,
bool isCurveAbove,
bool &discard,
int margin = 0,
376 bool horizontalOverlap =
true)
const;
378 std::pair<int, int> CalcLeftRightAdjustment(
379 const BoundingBox *boundingBox,
bool &discard,
int margin = 0,
bool horizontalOverlap =
true)
const;
380 std::pair<int, int> CalcDirectionalLeftRightAdjustment(
const BoundingBox *boundingBox,
bool isCurveAbove,
381 bool &discard,
int margin = 0,
bool horizontalOverlap =
true)
const;
387 void GetPoints(
Point points[4])
const;
389 int GetThickness()
const {
return m_thickness; }
390 curvature_CURVEDIR GetDir()
const {
return m_dir; }
396 bool HasCachedX12()
const;
398 std::pair<int, int> GetCachedX12()
const {
return m_cachedX12; }
399 void SetCachedX12(
const std::pair<int, int> &cachedX12) { m_cachedX12 = cachedX12; }
421 void SetCrossStaff(
Staff *crossStaff) { m_crossStaff = crossStaff; }
423 Staff *GetCrossStaff() {
return m_crossStaff; }
424 const Staff *GetCrossStaff()
const {
return m_crossStaff; }
425 bool IsCrossStaff()
const {
return m_crossStaff != NULL; }
431 void SetRequestedStaffSpace(
int space) { m_requestedStaffSpace = space; }
433 int GetRequestedStaffSpace()
const {
return m_requestedStaffSpace; }
453 curvature_CURVEDIR m_dir;
457 ArrayOfCurveSpannedElements m_spannedElements;
460 mutable int m_cachedMinMaxY;
463 std::pair<int, int> m_cachedX12;
468 int m_requestedStaffSpace;
483 m_boundingBox = NULL;
489 Point m_rotatedPoints[4];
FloatingObject * GetObject()
Getter for the FloatingObject (asserted, cannot be NULL)
Definition: floatingobject.h:200
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
void UpdateCurveParams(const Point points[4], int thickness, curvature_CURVEDIR curveDir)
Update the curve parameters.
int CalcAdjustment(const BoundingBox *boundingBox, bool &discard, int margin=0, bool horizontalOverlap=true) const
Calculate the adjustment needed for an element for the curve not to overlap with it.
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
char m_spanningType
The spanning type of the positioner for spanning control elements.
Definition: floatingobject.h:319
int GetSpaceBelow(const Doc *doc, const StaffAlignment *staffAlignment, const BoundingBox *horizOverlappingBBox) const
Calculate the vertical space below the element and above the bounding box.
bool HasHorizontalOverlapWith(const BoundingBox *bbox, int unit) const
Check for horizontal overlap with special consideration for extender lines.
This class represents a basic object.
Definition: object.h:59
This class represents a basic object for a curve (slur, tie) in the layout domain.
Definition: floatingobject.h:329
virtual bool IsCloserToStaffThan(const FloatingObject *other, data_STAFFREL drawingPlace) const
Check whether the current object must be positioned closer to the staff than the other.
Definition: floatingobject.h:108
FloatingObject * m_object
A pointer to the FloatingObject it represents.
Definition: floatingobject.h:306
char GetSpanningType() const
Getter for the spanning type.
Definition: floatingobject.h:215
void AdjustExtenders()
Align extender elements across systems.
std::pair< int, int > CalcRequestedStaffSpace(const StaffAlignment *alignment) const
Calculate the requested staff space above and below.
std::pair< int, bool > GetVerticalContentBoundaryRel(const Doc *doc, const BoundingBox *horizOverlappingBBox, bool contentTop) const
Determine the vertical content boundary.
void CalcDrawingYRel(const Doc *doc, const StaffAlignment *staffAlignment, const BoundingBox *horizOverlappingBBox)
Update the Y drawing relative position based on collision detection with the overlapping bounding box...
Simple class for representing bezier cCurve.
Definition: devicecontextbase.h:258
int m_drawingXRel
The X drawing relative position of the object.
Definition: floatingobject.h:291
Definition: floatingobject.h:475
StaffAlignment * m_alignment
A pointer to the StaffAlignment that owns it.
Definition: floatingobject.h:311
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
void Reset() override
Reset the object, that is 1) removing all children and 2) resetting all attributes.
bool HasVerticalContentOverlap(const Doc *doc, const BoundingBox *horizOverlappingBBox, int margin) const
Version of Boundary::VerticalContentOverlap which takes refined boundaries into account.
This class represents elements appearing within a measure.
Definition: floatingobject.h:28
This abstract class is the base class for all const functors.
Definition: functor.h:126
void AddSpannedElement(CurveSpannedElement *spannedElement)
Add a CurveSpannedElement to the FloatingCurvePositioner.
Definition: floatingobject.h:411
int CalcMinMaxY(const Point points[4]) const
Calculate the min or max Y for a set of points.
int GetAdmissibleHorizOverlapMargin(const BoundingBox *bbox, int unit) const
Return the horizontal margin for overlap with another element This can be negative,...
int m_drawingYRel
The Y drawing relative position of the object.
Definition: floatingobject.h:296
int m_drawingExtenderWidth
The horizontal width of the extender line whenever it is not included in the bounding box.
Definition: floatingobject.h:301
virtual bool IsExtenderElement() const
Check whether current object represents initial element or extender lines.
Definition: floatingobject.h:103
virtual std::pair< int, bool > GetVerticalContentBoundaryRel(const Doc *doc, const FloatingPositioner *positioner, const BoundingBox *horizOverlappingBBox, bool contentTop) const
Determine the vertical content boundary.
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
This class represents a basic object in the layout domain.
Definition: boundingbox.h:32
This class stores an alignement position staves will point to.
Definition: verticalaligner.h:172
void ClearSpannedElements()
Deletes all the CurveSpannedElement objects.
FloatingPositioner * GetCorrespFloatingPositioner(const FloatingObject *object)
Look for the FloatingPositioner corresponding to the current one but for another object.
Simple class for representing points.
Definition: devicecontextbase.h:203
void ResetCurveParams()
Reset the curve parameters in FloatingCurvePositioner::FloatingCurvePositioner and in FloatingCurvePo...
StaffAlignment * GetAlignment()
Getter for the StaffAlignment (asserted, cannot be NULL)
Definition: floatingobject.h:208
void MoveFrontHorizontal(int distance)
Moves bounding points horizontally or vertically by a specified distance.
This class represents a basic object in the layout domain.
Definition: floatingobject.h:165
const ArrayOfCurveSpannedElements * GetSpannedElements()
Return a const pointer to the spanned elements.
Definition: floatingobject.h:416