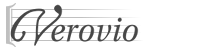 |
Verovio
Source code documentation
|
8 #ifndef __VRV_FTREM_H__
9 #define __VRV_FTREM_H__
12 #include "atts_shared.h"
14 #include "layerelement.h"
35 void Reset()
override;
36 std::string GetClassName()
const override {
return "fTrem"; }
42 BeamDrawingInterface *GetBeamDrawingInterface()
override {
return vrv_cast<BeamDrawingInterface *>(
this); }
44 const BeamDrawingInterface *GetBeamDrawingInterface()
const override
46 return vrv_cast<const BeamDrawingInterface *>(
this);
59 const ArrayOfBeamElementCoords *GetElementCoords();
78 FunctorCode
Accept(Functor &functor)
override;
80 FunctorCode
Accept(ConstFunctor &functor)
const override;
81 FunctorCode AcceptEnd(Functor &functor)
override;
82 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
91 void FilterList(ListOfConstObjects &childList)
const override;
95 BeamSegment m_beamSegment;
Object * Clone() const override
Method call for copying child classes.
Definition: ftrem.h:34
This class models the MEI <fTrem> element.
Definition: ftrem.h:25
This class represents a basic object.
Definition: object.h:59
void FilterList(ListOfConstObjects &childList) const override
Filter the flat list and keep only Note or Chords elements.
std::pair< int, int > GetAdditionalBeamCount() const override
See DrawingInterface::GetAdditionalBeamCount.
This class is an interface for MEI beam elements (beam, beamSpan).
Definition: drawinginterface.h:97
std::pair< int, int > GetFloatingBeamCount() const override
See DrawingInterface::GetFloatingBeamCount.
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
bool IsSupportedChild(ClassId classId) override
Add an element (a note or a chord) to a fTrem.