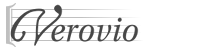 |
Verovio
Source code documentation
|
8 #ifndef __VRV_GLYPH_H__
9 #define __VRV_GLYPH_H__
16 #include "devicecontextbase.h"
38 Glyph(std::string path, std::string codeStr);
39 Glyph(
int unitsPerEm);
56 int GetUnitsPerEm()
const {
return m_unitsPerEm; }
58 void SetUnitsPerEm(
int units) { m_unitsPerEm = units; }
64 std::string GetCodeStr()
const {
return m_codeStr; }
66 void SetCodeStr(
const std::string &codeStr) { m_codeStr = codeStr; }
72 std::string GetPath()
const {
return m_path; }
74 void SetPath(
const std::string &path) { m_path = path; }
80 int GetHorizAdvX()
const {
return m_horizAdvX; }
82 void SetHorizAdvX(
double horizAdvX) { m_horizAdvX = (int)(horizAdvX * 10.0); }
88 bool GetFallback()
const {
return m_isFallback; }
90 void SetFallback(
bool isFallback) { m_isFallback = isFallback; }
97 void SetAnchor(std::string anchorStr,
double x,
double y);
102 bool HasAnchor(SMuFLGlyphAnchor anchor)
const;
113 void SetXML(
const std::string &xml) { m_xml = xml; }
119 std::string
GetXML()
const;
136 std::string m_codeStr;
142 std::map<SMuFLGlyphAnchor, Point> m_anchors;
void SetBoundingBox(double x, double y, double w, double h)
Set the bounds of the glyph These are original values from the font and will be stored as (int)(10....
const Point * GetAnchor(SMuFLGlyphAnchor anchor) const
Return the SMuFL anchor for the glyph.
bool HasAnchor(SMuFLGlyphAnchor anchor) const
Check if the glyph has anchor provided.
void SetAnchor(std::string anchorStr, double x, double y)
Add an anchor for the glyph.
void SetXML(const std::string &xml)
Set the XML (content) of the glyph.
Definition: glyph.h:113
This class is used for storing a music font glyph.
Definition: glyph.h:31
std::string GetXML() const
Return the XML (content) of the glyph.
Simple class for representing points.
Definition: devicecontextbase.h:203
void GetBoundingBox(int &x, int &y, int &w, int &h) const
Get the bounds of the glyph.