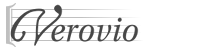 |
Verovio
Source code documentation
|
8 #ifndef __VRV_IOBASE_H__
9 #define __VRV_IOBASE_H__
69 void SetOutputFormat(
const std::string &format) { m_outformat = format; }
70 std::string GetOutputFormat() {
return m_outformat; }
73 virtual bool Import(std::string
const &data) {
return true; }
100 std::string m_outformat =
"mei";
virtual bool WriteObject(Object *object)
Dummy object method that must be overridden in child class.
Definition: iobase.h:42
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
This class represents a basic object.
Definition: object.h:59
This class is a base class for output classes.
Definition: iobase.h:31
virtual bool WriteObjectEnd(Object *object)
Dummy object method that must be overridden in child class.
Definition: iobase.h:47