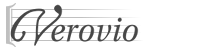 |
Verovio
Source code documentation
|
8 #ifndef __VRV_LAYER_ELEMENT_H__
9 #define __VRV_LAYER_ELEMENT_H__
15 #include "atts_shared.h"
16 #include "facsimileinterface.h"
18 #include "linkinginterface.h"
24 class BeamElementCoord;
33 struct AlignMeterParams;
36 enum StaffSearch { ANCESTOR_ONLY = 0, RESOLVE_CROSS_STAFF };
61 void Reset()
override;
72 FacsimileInterface *GetFacsimileInterface()
override {
return vrv_cast<FacsimileInterface *>(
this); }
76 return vrv_cast<const FacsimileInterface *>(
this);
78 LinkingInterface *GetLinkingInterface()
override {
return vrv_cast<LinkingInterface *>(
this); }
79 const LinkingInterface *GetLinkingInterface()
const override {
return vrv_cast<const LinkingInterface *>(
this); }
113 ElementScoreDefRole GetScoreDefRole()
const {
return m_scoreDefRole; }
115 void SetScoreDefRole(ElementScoreDefRole scoreDefRole) { m_scoreDefRole = scoreDefRole; }
137 bool IsInBeam()
const;
143 void SetDrawingCueSize(
bool drawingCueSize) {
m_drawingCueSize = drawingCueSize; }
151 void SetIsInBeamSpan(
bool isInBeamSpan);
153 bool GetIsInBeamSpan()
const {
return m_isInBeamspan; }
159 int GetAlignmentLayerN()
const {
return m_alignmentLayerN; }
161 void SetAlignmentLayerN(
int alignmentLayerN) { m_alignmentLayerN = alignmentLayerN; }
172 int GetDrawingX()
const override;
174 int GetDrawingY()
const override;
182 virtual void SetDrawingXRel(
int drawingXRel);
183 void CacheXRel(
bool restore =
false);
185 virtual void SetDrawingYRel(
int drawingYRel);
186 void CacheYRel(
bool restore =
false);
201 int GetDrawingTop(
const Doc *doc,
int staffSize,
bool withArtic =
true, ArticType articType = ARTIC_INSIDE)
const;
202 int GetDrawingBottom(
203 const Doc *doc,
int staffSize,
bool withArtic =
true, ArticType articType = ARTIC_INSIDE)
const;
215 const Alignment *
GetAlignment()
const {
return m_alignment; }
216 void ResetAlignment() { m_alignment = NULL; }
217 void SetAlignment(Alignment *alignment) { m_alignment = alignment; }
223 Staff *
GetAncestorStaff(StaffSearch strategy = ANCESTOR_ONLY,
bool assertExistence =
true);
225 const Staff *
GetAncestorStaff(StaffSearch strategy = ANCESTOR_ONLY,
bool assertExistence =
true)
const;
253 Alignment *GetGraceAlignment();
255 const Alignment *GetGraceAlignment()
const;
257 void SetGraceAlignment(Alignment *graceAlignment);
265 data_NOTATIONTYPE notationType = NOTATIONTYPE_cmn)
const;
271 Fraction
GetAlignmentDuration(
bool notGraceOnly =
true, data_NOTATIONTYPE notationType = NOTATIONTYPE_cmn)
const;
278 data_NOTATIONTYPE notationType = NOTATIONTYPE_cmn)
const;
280 Fraction GetContentAlignmentDuration(
const AlignMeterParams ¶ms,
bool notGraceOnly =
true,
281 data_NOTATIONTYPE notationType = NOTATIONTYPE_cmn)
const;
283 Fraction GetContentAlignmentDuration(
284 bool notGraceOnly =
true, data_NOTATIONTYPE notationType = NOTATIONTYPE_cmn)
const;
297 bool areDotsAdjusted,
bool &isUnison,
bool &stemSameAs);
304 bool areDotsAdjusted,
bool isChordElement,
bool isLowerElement =
false,
bool unison =
true);
337 FunctorCode AcceptEnd(
Functor &functor)
override;
338 FunctorCode AcceptEnd(
ConstFunctor &functor)
const override;
349 const std::set<int> &firstChord,
const std::set<int> &secondChord, data_STEMDIRECTION stemDirection)
const;
371 static int GetDotCount(
const MapOfDotLocs &dotLocations);
376 static int GetCollisionCount(
const MapOfDotLocs &dotLocs1,
const MapOfDotLocs &dotLocs2);
379 int GetDrawingArticulationTopOrBottom(data_STAFFREL place, ArticType type)
const;
436 ElementScoreDefRole m_scoreDefRole;
442 int m_alignmentLayerN;
void CenterDrawingX()
Adjust the m_drawingYRel for the element to be centered on the inner content of the measure.
Fraction GetSameAsContentAlignmentDuration(const AlignMeterParams ¶ms, bool notGraceOnly=true, data_NOTATIONTYPE notationType=NOTATIONTYPE_cmn) const
Return the duration if the content of the layer element with a @sameas attribute.
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
int m_cachedYRel
The cached value for m_drawingYRel for caching horizontal layout.
Definition: layerelement.h:414
int m_drawingXRel
The X drawing relative position of the object.
Definition: layerelement.h:420
Alignment * GetAlignment()
Alignment setter and getter.
Definition: layerelement.h:214
void CloneReset() override
Overriding CloneReset() method to be called after copy / assignment calls.
int GetDrawingRadius(const Doc *doc, bool isInLigature=false) const
Return the drawing radius for notes and chords.
bool IsInLigature() const
Return true if the element is a note within a ligature.
int m_drawingYRel
The Y drawing relative position of the object.
Definition: layerelement.h:409
FTrem * GetAncestorFTrem()
Return the FTrem parten if the element is a note or a chord within a fTrem.
Staff * GetCrossStaff(Layer *&layer)
Look for a cross or a a parent LayerElement (note, chord, rest) with a cross staff.
std::pair< int, bool > CalcElementHorizontalOverlap(const Doc *doc, const std::vector< LayerElement * > &otherElements, bool areDotsAdjusted, bool isChordElement, bool isLowerElement=false, bool unison=true)
Calculate note horizontal overlap with elemenents from another layers.
This class represents a basic object.
Definition: object.h:59
This class is an interface for elements having a link It is not an abstract class but should not be i...
Definition: linkinginterface.h:31
int m_cachedXRel
The cached value for m_drawingXRel for caching horizontal layout.
Definition: layerelement.h:425
static int GetDotCount(const MapOfDotLocs &dotLocations)
Helper to count the number of dots This can be used as an indicator to choose between different sets ...
Alignment * m_graceAlignment
An alignment for grace notes.
Definition: layerelement.h:403
int GetDrawingTop(const Doc *doc, int staffSize, bool withArtic=true, ArticType articType=ARTIC_INSIDE) const
Returns the drawing top and bottom taking into accound stem, etc.
virtual MapOfDotLocs CalcDotLocations(int layerCount, bool primary) const
The dot locations w.r.t.
Definition: layerelement.h:361
int GetOriginalLayerN() const
Staff * m_crossStaff
This stores a pointer to the cross-staff (if any) and the appropriate layer See PrepareCrossStaffFunc...
Definition: layerelement.h:394
virtual MapOfNoteLocs CalcNoteLocations(NotePredicate predicate=NULL) const
The note locations w.r.t.
Definition: layerelement.h:354
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
bool GenerateZoneBounds(int *ulx, int *uly, int *lrx, int *lry) const
Get zone bounds using child elements with facsimile information.
int m_drawingFacsX
Absolute position X.
Definition: layerelement.h:388
This class stores an alignment position elements will point to.
Definition: horizontalaligner.h:73
virtual bool HasCrossStaff() const
Return true if cross-staff is set.
Definition: layerelement.h:314
virtual bool IsRelativeToStaff() const
Return true if the element is relative to the staff and not to its parent.
Definition: layerelement.h:97
This abstract class is the base class for all const functors.
Definition: functor.h:126
static int GetCollisionCount(const MapOfDotLocs &dotLocs1, const MapOfDotLocs &dotLocs2)
Helper to count the collisions between two sets of dots.
virtual bool HasToBeAligned() const
Return true if the element has to be aligned horizontally It is typically set to false for mRest,...
Definition: layerelement.h:86
Definition: facsimileinterface.h:27
bool IsGraceNote() const
Return true if the element is a grace note.
data_STAFFREL_basic GetCrossStaffRel() const
Retrieve the direction of a cross-staff situation.
virtual int AdjustOverlappingLayers(const Doc *doc, const std::vector< LayerElement * > &otherElements, bool areDotsAdjusted, bool &isUnison, bool &stemSameAs)
Helper to adjust overlapping layers for notes, chords, stems, etc.
void GetOverflowStaffAlignments(StaffAlignment *&above, StaffAlignment *&below)
Get the StaffAlignment for which overflows need to be calculated against.
Fraction GetAlignmentDuration(const AlignMeterParams ¶ms, bool notGraceOnly=true, data_NOTATIONTYPE notationType=NOTATIONTYPE_cmn) const
Return the duration if the element has a DurationInterface.
virtual bool IsScoreDefElement() const
Return true if the element is part of a scoreDef or staffDef.
Definition: layerelement.h:91
MapOfDotLocs CalcOptimalDotLocations()
Calculate the optimal dot location for a note or chord Takes two layers into account in order to avoi...
bool m_drawingCueSize
The cached drawing cue size set by PrepareCueSizeFunctor.
Definition: layerelement.h:430
Beam * GetAncestorBeam()
Return the beam parent if in beam Look if the note or rest is in a beam.
void Reset() override
Virtual reset method.
virtual data_STEMMODIFIER GetDrawingStemMod() const
Get the stem mod for the element (if any)
Staff * GetAncestorStaff(StaffSearch strategy=ANCESTOR_ONLY, bool assertExistence=true)
Get the ancestor or cross staff.
std::vector< int > GetElementsInUnison(const std::set< int > &firstChord, const std::set< int > &secondChord, data_STEMDIRECTION stemDirection) const
Helper to figure whether two chords are in fully in unison based on the locations of the notes.
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
LayerElement * ThisOrSameasLink()
Return itself or the resolved @sameas (if any)
This class stores an alignement position staves will point to.
Definition: verticalaligner.h:172
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
This class represents a layer in a laid-out score (Doc).
Definition: layer.h:33
char32_t StemModToGlyph(data_STEMMODIFIER stemMod) const
Convert stem mode to corresponding glyph code.