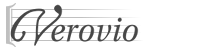 |
Verovio
Source code documentation
|
8 #ifndef __VRV_MEASURE_H__
9 #define __VRV_MEASURE_H__
12 #include "atts_shared.h"
14 #include "facsimileinterface.h"
15 #include "horizontalaligner.h"
43 public AttMeterConformanceBar,
44 public AttNNumberLike,
48 using BarlineRenditionPair = std::pair<data_BARRENDITION, data_BARRENDITION>;
55 Measure(MeasureType measuredMusic = MEASURED,
int logMeasureNb = -1);
59 void Reset()
override;
60 std::string GetClassName()
const override {
return "measure"; }
71 FacsimileInterface *GetFacsimileInterface()
override {
return vrv_cast<FacsimileInterface *>(
this); }
73 const FacsimileInterface *GetFacsimileInterface()
const override
75 return vrv_cast<const FacsimileInterface *>(
this);
87 bool IsNeumeLine()
const {
return (m_measureType == NEUMELINE); }
92 int GetIndex()
const {
return m_index; }
94 void SetIndex(
int index) { m_index = index; }
132 void SetDrawingXRel(
int drawingXRel);
133 void CacheXRel(
bool restore =
false);
141 bool IsFirstInSystem()
const;
143 bool IsLastInSystem()
const;
160 data_BARRENDITION GetDrawingLeftBarLine()
const {
return m_leftBarLine.GetForm(); }
162 void SetDrawingLeftBarLine(data_BARRENDITION type) { m_leftBarLine.SetForm(type); }
163 data_BARRENDITION GetDrawingRightBarLine()
const {
return m_rightBarLine.GetForm(); }
164 void SetDrawingRightBarLine(data_BARRENDITION type) { m_rightBarLine.SetForm(type); }
165 data_BARRENDITION GetDrawingLeftBarLineByStaffN(
int staffN)
const;
166 data_BARRENDITION GetDrawingRightBarLineByStaffN(
int staffN)
const;
191 Measure *previous, ListOfObjects ¤tInvisible, ListOfObjects &previousInvisible,
int barlineDrawingFlags);
199 BarLine *GetLeftBarLine() {
return &m_leftBarLine; }
201 const BarLine *GetLeftBarLine()
const {
return &m_leftBarLine; }
202 BarLine *GetRightBarLine() {
return &m_rightBarLine; }
203 const BarLine *GetRightBarLine()
const {
return &m_rightBarLine; }
214 int GetLeftBarLineXRel()
const;
216 int GetLeftBarLineLeft()
const;
217 int GetLeftBarLineRight()
const;
218 int GetRightBarLineXRel()
const;
219 int GetRightBarLineLeft()
const;
220 int GetRightBarLineRight()
const;
248 int GetCachedOverflow()
const {
return m_cachedOverflow; }
249 void ResetCachedWidth() { m_cachedWidth = VRV_UNSET; }
250 void ResetCachedOverflow() { m_cachedOverflow = VRV_UNSET; }
267 ScoreDef *GetDrawingScoreDef() {
return m_drawingScoreDef; }
269 const ScoreDef *GetDrawingScoreDef()
const {
return m_drawingScoreDef; }
270 void SetDrawingScoreDef(ScoreDef *drawingScoreDef);
271 void ResetDrawingScoreDef();
277 Ending *GetDrawingEnding() {
return m_drawingEnding; }
279 const Ending *GetDrawingEnding()
const {
return m_drawingEnding; }
280 void SetDrawingEnding(Ending *ending) { m_drawingEnding = ending; }
287 bool HasAlignmentRefWithMultipleLayers()
const {
return m_hasAlignmentRefWithMultipleLayers; }
289 void HasAlignmentRefWithMultipleLayers(
bool hasRef) { m_hasAlignmentRefWithMultipleLayers = hasRef; }
295 std::vector<Staff *> GetFirstStaffGrpStaves(ScoreDef *scoreDef);
331 double GetRealTimeOffsetMilliseconds(
int repeat)
const;
339 void AddScoreTimeOffset(Fraction offset) { m_scoreTimeOffset.push_back(offset); }
340 void ClearRealTimeOffset() { m_realTimeOffsetMilliseconds.clear(); }
341 void AddRealTimeOffset(
double milliseconds) { m_realTimeOffsetMilliseconds.push_back(milliseconds); }
349 double GetCurrentTempo()
const {
return m_currentTempo; }
364 FunctorCode
Accept(Functor &functor)
override;
366 FunctorCode
Accept(ConstFunctor &functor)
const override;
367 FunctorCode AcceptEnd(Functor &functor)
override;
368 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
373 enum BarlineDrawingFlags {
375 SCORE_DEF_INSERT = 0x2,
376 INVISIBLE_MEASURE_CURRENT = 0x4,
377 INVISIBLE_MEASURE_PREVIOUS = 0x8
410 int m_cachedOverflow;
420 MeasureType m_measureType;
451 bool m_hasAlignmentRefWithMultipleLayers;
456 std::vector<Fraction> m_scoreTimeOffset;
457 std::vector<double> m_realTimeOffsetMilliseconds;
458 double m_currentTempo;
460 std::map<int, BarlineRenditionPair> m_invisibleStaffBarlines;
Staff * GetBottomVisibleStaff()
Return the bottom (last) visible staff in the measure (if any).
This class represents a measure in a page-based score (Doc).
Definition: measure.h:37
void SetCurrentTempo(double tempo)
Setter and getter for the current tempo.
Definition: measure.h:348
bool IsSupportedChild(ClassId classId) override
Methods for adding allowed content.
This class stores the timestamps (TimestampsAttr) in a measure.
Definition: horizontalaligner.h:664
int GetMeasureIdx() const
Return the index position of the measure in its system parent.
Definition: measure.h:149
void SetDrawingBarLines(Measure *previous, int barlineDrawingFlags)
Set the drawing barlines for the measure.
void ClearScoreTimeOffset()
Setter for the time offset.
Definition: measure.h:338
int GetCachedWidth() const
Return and reset the cached width / overflow.
Definition: measure.h:247
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
void CloneReset() override
Overriding CloneReset() method to be called after copy / assignment calls.
This class represents a MEI ending.
Definition: ending.h:28
BarlineRenditionPair SelectDrawingBarLines(const Measure *previous) const
Select drawing barlines based on the previous right and current left barlines (to avoid duplicated do...
int CalculateRightBarLineWidth(const Doc *doc, int staffSize) const
Return the width of the right barline based on the barline form.
int GetIdx() const
Return the index position of the object in its parent (-1 if not found)
int GetInnerCenterX() const
Return the center x of the inner of the measure.
double GetLastRealTimeOffset() const
Return the real time offset in milliseconds.
Definition: measure.h:330
This class represents a basic object.
Definition: object.h:59
int GetNonJustifiableLeftMargin() const
Return the non-justifiable left margin for the measure.
Definition: measure.h:209
int m_drawingFacsX1
The X absolute position of the measure for facsimile (transcription) encodings.
Definition: measure.h:384
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
int EnclosesTime(int time) const
Check if the measure encloses the given time (in millisecond) Return the playing repeat time (1-based...
Definition: fraction.h:19
void SetInvisibleStaffBarlines(Measure *previous, ListOfObjects ¤tInvisible, ListOfObjects &previousInvisible, int barlineDrawingFlags)
Create mapping of original barline values to staves in the measure that are neighbored by invisible s...
void ResetCachedDrawingX() const override
Reset the cached values of the drawingX values.
int GetSectionRestartShift(const Doc *doc) const
Calculates the section restart shift.
This class models the MEI <barLine> element.
Definition: barline.h:29
std::vector< std::pair< LayerElement *, LayerElement * > > GetInternalTieEndpoints()
Return vector with tie endpoints for ties that start and end in current measure.
bool AddChildAdditionalCheck(Object *child) override
Additional check when adding a child.
int m_cachedXRel
The cached value for m_drawingXRel for caching horizontal layout.
Definition: measure.h:405
int GetDrawingOverflow()
Return the right overflow of the control events in the measure.
int GetInnerWidth() const
Return the inner width of the measure.
void AddChildBack(Object *object)
Specific method for measures.
This class aligns the content of a measure It contains a vector of Alignment.
Definition: horizontalaligner.h:425
int GetIndex() const
Get and set the measure index.
Definition: measure.h:93
void Reset() override
Virtual reset method.
Staff * GetTopVisibleStaff()
Return the top (first) visible staff in the measure (if any).
Definition: facsimileinterface.h:27
bool HasCachedHorizontalLayout() const
Return true if the Measure has cached values for the horizontal layout.
Definition: measure.h:115
bool IsMeasuredMusic() const
Return true if measured music (otherwise we have fake measures)
Definition: measure.h:82
int GetDrawingX() const override
Get the X drawing position.
Fraction GetLastTimeOffset() const
Read only access to m_scoreTimeOffset.
Definition: measure.h:324
bool IsNeumeLine() const
Return true if the measure represents a neume (section) line.
Definition: measure.h:87
int m_drawingXRel
The X relative position of the measure.
Definition: measure.h:400
This class represents a MEI scoreDef.
Definition: scoredef.h:129
MeasureAligner m_measureAligner
The measure aligner that holds the x positions of the content of the measure.
Definition: measure.h:391
int GetWidth() const
Return the width of the measure, including the barLine width.
bool HasInvisibleStaffBarlines() const
Return whether there is mapping of barline values to invisible staves present in measure.
Definition: measure.h:172
Object * Clone() const override
Method call for copying child classes.
Definition: measure.h:58