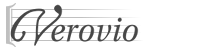 |
Verovio
Source code documentation
|
8 #ifndef __VRV_MISCFUNCTOR_H__
9 #define __VRV_MISCFUNCTOR_H__
41 FunctorCode VisitLayerElement(
LayerElement *layerElement)
override;
42 FunctorCode VisitMeasure(
Measure *measure)
override;
43 FunctorCode VisitPage(
Page *page)
override;
44 FunctorCode VisitStaff(
Staff *staff)
override;
45 FunctorCode VisitSurface(
Surface *surface)
override;
46 FunctorCode VisitSystem(
System *system)
override;
47 FunctorCode VisitZone(
Zone *zone)
override;
86 void ExcludeClasses(
const std::vector<ClassId> &excludeClasses) { m_excludeClasses = excludeClasses; }
92 int GetMinLeft()
const {
return m_minLeft; }
93 int GetMaxRight()
const {
return m_maxRight; }
100 FunctorCode VisitObject(
const Object *
object)
override;
114 std::vector<ClassId> m_excludeClasses;
143 const IntTree &GetLayerTree()
const {
return m_layerTree; }
144 const IntTree &GetVerseTree()
const {
return m_verseTree; }
151 FunctorCode VisitLayer(
const Layer *layer)
override;
152 FunctorCode VisitVerse(
const Verse *verse)
override;
194 FunctorCode VisitObject(
Object *
object)
override;
209 #endif // __VRV_MISCFUNCTOR_H__
This class represents a staff in a laid-out score (Doc).
Definition: staff.h:102
This class builds a tree of ints (IntTree) with the staff/layer/verse numbers.
Definition: miscfunctor.h:124
This class represents a measure in a page-based score (Doc).
Definition: measure.h:37
This class retrieves the minimum left and maximum right for an alignment.
Definition: miscfunctor.h:68
Implements the zone element in MEI.
Definition: zone.h:30
Implements the surface element in MEI.
Definition: surface.h:30
This class represents a basic object.
Definition: object.h:59
This class applies the Pixel Per Unit factor of the page to its elements.
Definition: miscfunctor.h:22
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: miscfunctor.h:35
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: miscfunctor.h:188
This class reorders elements by x-position.
Definition: miscfunctor.h:175
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: miscfunctor.h:137
Generic int map recursive structure for storing hierachy of values For example, we want to process al...
Definition: vrvdef.h:419
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
This class represents a page in a laid-out score (Doc).
Definition: page.h:31
This abstract class is the base class for all const functors.
Definition: functor.h:126
This class represents a system in a laid-out score (Doc).
Definition: system.h:36
bool ImplementsEndInterface() const override
Return true if the functor implements the end interface.
Definition: miscfunctor.h:81
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46