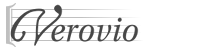 |
Verovio
Source code documentation
|
15 #include "atts_analytical.h"
16 #include "atts_neumes.h"
17 #include "atts_shared.h"
18 #include "atts_visual.h"
19 #include "durationinterface.h"
20 #include "layerelement.h"
21 #include "pitchinterface.h"
22 #include "positioninterface.h"
39 public AttCurvatureDirection,
40 public AttIntervalMelodic,
51 void Reset()
override;
52 std::string GetClassName()
const override {
return "nc"; }
60 DurationInterface *GetDurationInterface()
override {
return vrv_cast<DurationInterface *>(
this); }
62 const DurationInterface *GetDurationInterface()
const override {
return vrv_cast<const DurationInterface *>(
this); }
63 PitchInterface *GetPitchInterface()
override {
return vrv_cast<PitchInterface *>(
this); }
64 const PitchInterface *GetPitchInterface()
const override {
return vrv_cast<const PitchInterface *>(
this); }
65 PositionInterface *GetPositionInterface()
override {
return vrv_cast<PositionInterface *>(
this); }
66 const PositionInterface *GetPositionInterface()
const override {
return vrv_cast<const PositionInterface *>(
this); }
82 FunctorCode
Accept(Functor &functor)
override;
84 FunctorCode
Accept(ConstFunctor &functor)
const override;
85 FunctorCode AcceptEnd(Functor &functor)
override;
86 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
96 float m_xOffset = 0.0;
97 float m_yOffset = 0.0;
This class models the MEI <nc> element.
Definition: nc.h:34
This class is an interface for elements with a position on the staff, such as rests.
Definition: positioninterface.h:30
This class represents a basic object.
Definition: object.h:59
void Reset() override
Virtual reset method.
int PitchOrLocDifferenceTo(const Nc *nc) const
Calclulate the pitch or loc difference between to nc.
A Structure holding a glyph paramter for the nc.
Definition: nc.h:94
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
bool IsSupportedChild(ClassId classId) override
Base method for checking if a child can be added.
Object * Clone() const override
Method call for copying child classes.
Definition: nc.h:50
This class is an interface for elements with duration, such as notes and rests.
Definition: durationinterface.h:31
std::vector< DrawingGlyph > m_drawingGlyphs
Drawing glyphs.
Definition: nc.h:104
This class is an interface for elements with pitch, such as notes and neumes.
Definition: pitchinterface.h:28
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46