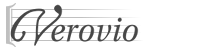 |
Verovio
Source code documentation
|
16 #include "altsyminterface.h"
17 #include "atts_analytical.h"
18 #include "atts_externalsymbols.h"
19 #include "atts_mensural.h"
20 #include "atts_midi.h"
21 #include "atts_shared.h"
22 #include "atts_stringtab.h"
25 #include "durationinterface.h"
26 #include "layerelement.h"
27 #include "pitchinterface.h"
28 #include "transposition.h"
57 public AttExtSymNames,
59 public AttHarmonicFunction,
60 public AttMidiVelocity,
62 public AttNoteVisMensural,
67 public AttVisibility {
77 void Reset()
override;
78 std::string GetClassName()
const override {
return "note"; }
84 AltSymInterface *GetAltSymInterface()
override {
return vrv_cast<AltSymInterface *>(
this); }
86 const AltSymInterface *GetAltSymInterface()
const override {
return vrv_cast<const AltSymInterface *>(
this); }
87 DurationInterface *GetDurationInterface()
override {
return vrv_cast<DurationInterface *>(
this); }
88 const DurationInterface *GetDurationInterface()
const override {
return vrv_cast<const DurationInterface *>(
this); }
89 PitchInterface *GetPitchInterface()
override {
return vrv_cast<PitchInterface *>(
this); }
90 const PitchInterface *GetPitchInterface()
const override {
return vrv_cast<const PitchInterface *>(
this); }
91 PositionInterface *GetPositionInterface()
override {
return vrv_cast<PositionInterface *>(
this); }
92 const PositionInterface *GetPositionInterface()
const override {
return vrv_cast<const PositionInterface *>(
this); }
93 StemmedDrawingInterface *GetStemmedDrawingInterface()
override {
return vrv_cast<StemmedDrawingInterface *>(
this); }
94 const StemmedDrawingInterface *GetStemmedDrawingInterface()
const override
96 return vrv_cast<const StemmedDrawingInterface *>(
this);
130 Accid *GetDrawingAccid();
132 const Accid *GetDrawingAccid()
const;
141 data_DURATION GetDrawingDur()
const;
142 bool IsNoteGroupExtreme()
const;
156 std::u32string GetTabFretString(data_NOTATIONTYPE notationType,
int &overline,
int &strike,
int &underline)
const;
169 void SetNoteGroup(ChordNoteGroup *noteGroup,
int position);
171 ChordNoteGroup *GetNoteGroup() {
return m_noteGroup; }
172 int GetNoteGroupPosition()
const {
return m_noteGroupPosition; }
178 void SetFlippedNotehead(
bool flippedNotehead) { m_flippedNotehead = flippedNotehead; }
180 bool GetFlippedNotehead()
const {
return m_flippedNotehead; }
192 Point
GetStemUpSE(
const Doc *doc,
int staffSize,
bool isCueSize)
const override;
194 Point GetStemDownNW(
const Doc *doc,
int staffSize,
bool isCueSize)
const override;
195 int CalcStemLenInThirdUnits(
const Staff *staff, data_STEMDIRECTION stemDir)
const override;
221 int GetMIDIPitch(
int shift = 0,
int octaveShift = 0)
const;
231 bool HasStemSameasNote()
const {
return (m_stemSameas); }
233 Note *GetStemSameasNote() {
return m_stemSameas; }
234 const Note *GetStemSameasNote()
const {
return m_stemSameas; }
235 void SetStemSameasNote(Note *stemSameas) { m_stemSameas = stemSameas; }
243 void SetStemSameasRole(StemSameasDrawingRole stemSameasRole) { m_stemSameasRole = stemSameasRole; }
282 void UpdateFromTransPitch(
const TransPitch &tp,
bool hasKeySig);
292 FunctorCode
Accept(Functor &functor)
override;
294 FunctorCode
Accept(ConstFunctor &functor)
const override;
295 FunctorCode AcceptEnd(Functor &functor)
override;
296 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
316 int GetChromaticAlteration()
const;
324 bool m_flippedNotehead;
329 ChordNoteGroup *m_noteGroup;
334 int m_noteGroupPosition;
350 StemSameasDrawingRole m_stemSameasRole;
365 bool operator()(
const Object *first,
const Object *second)
const
367 const Note *n1 =
dynamic_cast<const Note *
>(first);
368 const Note *n2 =
dynamic_cast<const Note *
>(second);
386 bool operator()(
const Object *first,
const Object *second)
const
388 const Note *n1 =
dynamic_cast<const Note *
>(first);
389 const Note *n2 =
dynamic_cast<const Note *
>(second);
391 return (n1->GetTabCourse() > n2->GetTabCourse());
int GetDiatonicPitch() const
Returns a single integer representing pitch and octave.
int GetPitchClass() const
Get pitch class of the current note.
void CalcNoteHeadShiftForSameasNote(Note *stemSameas, data_STEMDIRECTION stemDir)
Set the Note::m_flippedNotehead flag if one of the two notes needs to be placed on the side.
This class is an interface for elements with a position on the staff, such as rests.
Definition: positioninterface.h:30
This class represents a collection of notes in the same layer with the same onset time.
Definition: chord.h:32
This class models the MEI <accid> element.
Definition: accid.h:27
bool IsSupportedChild(ClassId classId) override
Add an element (a verse or an accid) to a note.
This class represents a basic object.
Definition: object.h:59
TabGrp * IsTabGrpNote()
Return the parent TabGrp is the note is part of one.
data_STEMDIRECTION CalcStemDirForSameasNote(int verticalCenter)
Calculate the stem direction of the pair of notes.
Chord * IsChordTone()
Overriding functions to return information from chord parent if any.
char32_t GetNoteheadGlyph(const data_DURATION duration) const
Return a SMuFL code for the notehead.
Unary predicate for sorting notes by course number.
Definition: note.h:381
This class models the MEI <tabGrp> element.
Definition: tabgrp.h:23
bool IsVisible() const
Check if a note or its parent chord are visible.
bool HasToBeAligned() const override
Override the method since alignment is required.
Definition: note.h:104
StemSameasDrawingRole GetStemSameasRole() const
Getter and setter for stem sameas role.
Definition: note.h:242
bool AddChildAdditionalCheck(Object *child) override
Additional check when adding a child.
This class is an interface for elements having a @altsym It is not an abstract class but should not b...
Definition: altsyminterface.h:30
MapOfNoteLocs CalcNoteLocations(NotePredicate predicate=NULL) const override
The note locations w.r.t.
bool IsUnisonWith(const Note *note, bool ignoreAccid=false) const
Return true if the note is a unison.
Point GetStemUpSE(const Doc *doc, int staffSize, bool isCueSize) const override
Get the stem up / stem down attachment point.
This class models the MEI <note> element.
Definition: note.h:47
char32_t GetMensuralNoteheadGlyph() const
Return the SMuFL code for a mensural note looking at the staff notation type, the coloration and the ...
bool IsEnharmonicWith(const Note *note) const
Check whether current note is enharmonic with another.
Object * Clone() const override
Method call for copying child classes.
Definition: note.h:76
Unary predicate for sorting notes by diatonic pitch.
Definition: note.h:360
void AddChild(Object *object) override
Overwritten method for note.
MapOfDotLocs CalcDotLocations(int layerCount, bool primary) const override
The dot locations w.r.t.
static bool HandleLedgerLineStemCollision(const Doc *doc, const Staff *staff, const Note *note1, const Note *note2)
Assume that two notes from different layers are given occuring at the same time Returns true if one n...
int GetMIDIPitch(int shift=0, int octaveShift=0) const
MIDI pitch.
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
TransPitch GetTransPitch() const
Get and set the pitch for transposition.
This class is an interface for MEI stemmed element.
Definition: drawinginterface.h:353
void AlignDotsShift(const Note *otherNote)
Align dots shift for two notes.
This class is an interface for elements with duration, such as notes and rests.
Definition: durationinterface.h:31
This class is an interface for elements with pitch, such as notes and neumes.
Definition: pitchinterface.h:28
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
void Reset() override
Virtual reset method.
static int PnameToPclass(data_PITCHNAME pitchName)
Get pitch class based on the pitch name.