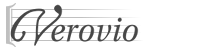 |
Verovio
Source code documentation
|
8 #ifndef __VRV_SCOREDEF_H__
9 #define __VRV_SCOREDEF_H__
11 #include "atts_gestural.h"
13 #include "atts_shared.h"
14 #include "drawinginterface.h"
16 #include "scoredefinterface.h"
51 void Reset()
override;
57 ScoreDefInterface *GetScoreDefInterface()
override {
return vrv_cast<ScoreDefInterface *>(
this); }
59 const ScoreDefInterface *GetScoreDefInterface()
const override {
return vrv_cast<const ScoreDefInterface *>(
this); }
65 bool HasClefInfo(
int depth = 1)
const;
67 bool HasKeySigInfo(
int depth = 1)
const;
68 bool HasMensurInfo(
int depth = 1)
const;
69 bool HasMeterSigInfo(
int depth = 1)
const;
70 bool HasMeterSigGrpInfo(
int depth = 1)
const;
83 const Clef *GetClef()
const;
84 Clef *GetClefCopy()
const;
86 const KeySig *GetKeySig()
const;
87 KeySig *GetKeySigCopy()
const;
89 const Mensur *GetMensur()
const;
90 Mensur *GetMensurCopy()
const;
109 FunctorCode AcceptEnd(
Functor &functor)
override;
110 FunctorCode AcceptEnd(
ConstFunctor &functor)
const override;
133 public AttOptimization,
145 void Reset()
override;
146 std::string GetClassName()
const override {
return "scoreDef"; }
201 const StaffGrp *
GetStaffGrp(
const std::string &n)
const;
218 bool DrawLabels()
const {
return m_drawLabels; }
220 void SetDrawLabels(
bool drawLabels) { m_drawLabels = drawLabels; }
226 int GetDrawingWidth()
const {
return m_drawingWidth; }
228 void SetDrawingWidth(
int drawingWidth);
234 int GetDrawingLabelsWidth()
const {
return m_drawingLabelsWidth; }
236 void SetDrawingLabelsWidth(
int width);
237 void ResetDrawingLabelsWidth() { m_drawingLabelsWidth = 0; }
243 PgFoot *GetPgFoot(data_PGFUNC func);
245 const PgFoot *GetPgFoot(data_PGFUNC func)
const;
246 PgHead *GetPgHead(data_PGFUNC func);
247 const PgHead *GetPgHead(data_PGFUNC func)
const;
255 bool IsSectionRestart()
const;
269 FunctorCode
Accept(Functor &functor)
override;
271 FunctorCode
Accept(ConstFunctor &functor)
const override;
272 FunctorCode AcceptEnd(Functor &functor)
override;
273 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
280 void FilterList(ListOfConstObjects &childList)
const override;
286 bool m_insertScoreDef;
294 int m_drawingLabelsWidth;
void SetRedrawFlags(int redrawFlags)
Set the redraw flag to all staffDefs.
void FilterList(ListOfConstObjects &childList) const override
Filter the flat list and keep only StaffDef elements.
This class models the MEI <meterSig> element.
Definition: metersig.h:27
StaffGrp * GetStaffGrp(const std::string &n)
Get the staffGrp with number n (NULL if not found).
std::vector< int > GetStaffNs() const
Return all the @n values of the staffDef in a scoreDef.
This class is a base class for MEI scoreDef or staffDef elements.
Definition: scoredef.h:42
This class represents a basic object.
Definition: object.h:59
void ReplaceDrawingValues(const ScoreDef *newScoreDef)
Replace the scoreDef with the content of the newScoreDef.
void Reset() override
Reset the object, that is 1) removing all children and 2) resetting all attributes.
void Reset() override
Reset the object, that is 1) removing all children and 2) resetting all attributes.
This class represents a MEI meterSigGrp.
Definition: metersiggrp.h:28
This class models the MEI <mensur> element.
Definition: mensur.h:27
This class is an interface for elements implementing score attributes, such as <scoreDef>,...
Definition: scoredefinterface.h:31
bool AddChildAdditionalCheck(Object *child) override
Additional check when adding a child.
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
void ReplaceDrawingLabels(const StaffGrp *newStaffGrp)
Replace the corresponding staffGrp with the labels of the newStaffGrp.
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
bool HasSystemStartLine() const
void ResetFromDrawingValues()
Replace the staffDef score attributes with the ones currently set as drawing values.
This class models the MEI <clef> element.
Definition: clef.h:27
This abstract class is the base class for all mutable functors.
Definition: functor.h:101
This class is an pseudo interface for elements maintaining a flat list of children LayerElement for p...
Definition: object.h:873
This abstract class is the base class for all const functors.
Definition: functor.h:126
StaffDef * GetStaffDef(int n)
Get the staffDef with number n (NULL if not found).
int GetInsertOrderFor(ClassId classId) const override
Return an order for the given ClassId.
bool IsSupportedChild(ClassId classId) override
Check if a object is allowed as child.
This class represents a MEI scoreDef.
Definition: scoredef.h:129
This class models the MEI <keySig> element.
Definition: keysig.h:44
int GetMaxStaffSize() const
Return the maximum staff size in the scoreDef (100 if empty)
Object * Clone() const override
Method call for copying child classes.
Definition: scoredef.h:144