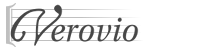 |
Verovio
Source code documentation
|
11 #include "atts_shared.h"
12 #include "facsimileinterface.h"
13 #include "layerelement.h"
14 #include "timeinterface.h"
46 void Reset()
override;
47 std::string GetClassName()
const override {
return "syl"; }
56 TimePointInterface *GetTimePointInterface()
override {
return vrv_cast<TimePointInterface *>(
this); }
58 const TimePointInterface *GetTimePointInterface()
const override
60 return vrv_cast<const TimePointInterface *>(
this);
62 TimeSpanningInterface *GetTimeSpanningInterface()
override {
return vrv_cast<TimeSpanningInterface *>(
this); }
63 const TimeSpanningInterface *GetTimeSpanningInterface()
const override
65 return vrv_cast<const TimeSpanningInterface *>(
this);
85 int GetDrawingWidth()
const;
86 int GetDrawingHeight()
const;
104 FunctorCode
Accept(Functor &functor)
override;
106 FunctorCode
Accept(ConstFunctor &functor)
const override;
107 FunctorCode AcceptEnd(Functor &functor)
override;
108 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
bool CreateDefaultZone(Doc *doc)
Create a default zone for a syl based on syllable.
int CalcHyphenLength(Doc *doc, int staffSize)
Calculate the hyphen length using the text font.
int m_drawingVerseN
The verse number with multiple verses Value is 1 by default, set in PrepareLyrics.
Definition: syl.h:121
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
This class represents a basic object.
Definition: object.h:59
void Reset() override
Virtual reset method.
bool IsSupportedChild(ClassId classId) override
Add an element (text, rend.
int CalcConnectorSpacing(Doc *doc, int staffSize)
Calculate the spacing needed depending on the @worpos and @con.
Syl * m_nextWordSyl
A pointer to the next syllable of the word.
Definition: syl.h:129
static void AdjustToLyricSize(const Doc *doc, int &value)
Adjust proportionally to the lyric size.
This class is an pseudo interface for elements maintaining a flat list of children LayerElement for p...
Definition: object.h:972
data_STAFFREL m_drawingVersePlace
The verse place (below by default)
Definition: syl.h:123
Object * Clone() const override
Method call for copying child classes.
Definition: syl.h:45
Syl is a TimeSpanningInterface for managing syllable connectors.
Definition: syl.h:31
This class is an interface for spanning elements, such as slur, hairpin, etc.
Definition: timeinterface.h:141
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
This class is an interface for elements having a single time point, such as tempo,...
Definition: timeinterface.h:35
bool IsRelativeToStaff() const override
Override the method since it is align to the staff.
Definition: syl.h:51