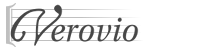 |
Verovio
Source code documentation
|
8 #ifndef __VRV_SYSTEM_H__
9 #define __VRV_SYSTEM_H__
11 #include "drawinginterface.h"
12 #include "editorial.h"
14 #include "verticalaligner.h"
19 class SystemMilestoneEnd;
45 void Reset()
override;
46 std::string GetClassName()
const override {
return "system"; }
59 int GetDrawingX()
const override;
61 int GetDrawingY()
const override;
69 virtual void SetDrawingXRel(
int drawingXRel);
71 virtual void SetDrawingYRel(
int drawingYRel);
77 int GetDrawingLabelsWidth()
const;
79 void SetDrawingLabelsWidth(
int width);
81 void SetDrawingAbbrLabelsWidth(
int width);
100 bool SetCurrentFloatingPositioner(
106 ScoreDef *GetDrawingScoreDef() {
return m_drawingScoreDef; }
108 const ScoreDef *GetDrawingScoreDef()
const {
return m_drawingScoreDef; }
109 void SetDrawingScoreDef(ScoreDef *drawingScoreDef);
110 void ResetDrawingScoreDef();
123 const LayerElement *start,
const LayerElement *end,
const Slur *slur)
const;
128 bool IsDrawingOptimized()
const {
return m_drawingIsOptimized; }
130 void IsDrawingOptimized(
bool drawingIsOptimized) { m_drawingIsOptimized = drawingIsOptimized; }
143 bool IsFirstInPage()
const;
145 bool IsLastInPage()
const;
146 bool IsFirstOfMdiv()
const;
147 bool IsLastOfMdiv()
const;
148 bool IsFirstOfSelection()
const;
149 bool IsLastOfSelection()
const;
169 FunctorCode
Accept(Functor &functor)
override;
171 FunctorCode
Accept(ConstFunctor &functor)
const override;
172 FunctorCode AcceptEnd(Functor &functor)
override;
173 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
177 SystemAligner m_systemAligner;
203 int m_drawingTotalWidth;
205 int m_drawingJustifiableWidth;
212 int m_castOffTotalWidth;
214 int m_castOffJustifiableWidth;
239 bool m_drawingIsOptimized;
int GetMinimumSystemSpacing(const Doc *doc) const
Return the minimus system spacing.
int m_systemLeftMar
System left margin (MEI scoredef@system.leftmar).
Definition: system.h:181
int GetSystemIdx() const
Return the index position of the system in its page parent.
Definition: system.h:98
This class is a hold the data and corresponds to the model of a MVC design pattern.
Definition: doc.h:41
int m_drawingFacsY
The Y absolute position of the staff for facsimile (transcription) encodings.
Definition: system.h:188
double EstimateJustificationRatio(const Doc *doc) const
Estimate the justification ratio from the castoff system widths and the desired page width.
int GetIdx() const
Return the index position of the object in its parent (-1 if not found)
This class is an interface for elements with duration, such as notes and rests.
Definition: drawinginterface.h:33
This class represents a basic object.
Definition: object.h:59
int m_drawingAbbrLabelsWidth
The width used by the abbreviated labels at the left of the system.
Definition: system.h:198
int m_drawingXRel
The X relative position of the system.
Definition: system.h:222
int GetHeight() const
Return the height of the system.
int m_drawingFacsX
The x absolute position of the system for facsimile layouts.
Definition: system.h:193
curvature_CURVEDIR GetPreferredCurveDirection(const LayerElement *start, const LayerElement *end, const Slur *slur) const
Get preferred curve direction based on the starting and ending point of the slur.
void AddToDrawingListIfNecessary(Object *object)
Add an object to the drawing list but only if necessary.
This class represents elements appearing within a measure.
Definition: floatingobject.h:28
int m_systemRightMar
System right margin (MEI scoredef@system.rightmar).
Definition: system.h:183
This class represents a system in a laid-out score (Doc).
Definition: system.h:36
This class represents a MEI scoreDef.
Definition: scoredef.h:129
bool IsSupportedChild(ClassId classId) override
Base method for checking if a child can be added.
bool HasMixedDrawingStemDir(const LayerElement *start, const LayerElement *end) const
Check if the notes between the start and end have mixed drawing stem directions.
int m_drawingYRel
The Y relative position of the system.
Definition: system.h:227
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
void ConvertToUnCastOffMensuralSystem()
Reverse of ConvertToCastOffMensural()