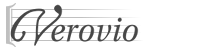 |
Verovio
Source code documentation
|
12 #include "atts_cmnornaments.h"
13 #include "atts_externalsymbols.h"
14 #include "controlelement.h"
15 #include "timeinterface.h"
29 public AttExtSymNames,
30 public AttOrnamentAccid,
31 public AttPlacementRelStaff,
42 void Reset()
override;
43 std::string GetClassName()
const override {
return "turn"; }
49 TimePointInterface *GetTimePointInterface()
override {
return vrv_cast<TimePointInterface *>(
this); }
51 const TimePointInterface *GetTimePointInterface()
const override
53 return vrv_cast<const TimePointInterface *>(
this);
74 FunctorCode
Accept(Functor &functor)
override;
76 FunctorCode
Accept(ConstFunctor &functor)
const override;
77 FunctorCode AcceptEnd(Functor &functor)
override;
78 FunctorCode AcceptEnd(ConstFunctor &functor)
const override;
This class models the MEI <turn> element.
Definition: turn.h:26
This class represents a basic object.
Definition: object.h:59
void Reset() override
Virtual reset method.
char32_t GetTurnGlyph() const
Get the SMuFL glyph for the turn based on form or glyph.num.
Object * Clone() const override
Method call for copying child classes.
Definition: turn.h:41
FunctorCode Accept(Functor &functor) override
Interface for class functor visitation.
LayerElement * m_drawingEndElement
The end point of a delayed turn when @startid is used.
Definition: turn.h:89
This class represents elements appearing within a measure.
Definition: controlelement.h:28
This class is a base class for the Layer (<layer>) content.
Definition: layerelement.h:46
int GetTurnHeight(const Doc *doc, int staffSize) const
Get the turn height ignoring slash.
This class is an interface for elements having a single time point, such as tempo,...
Definition: timeinterface.h:35